Comparing 5 Multithreaded Embedded Databases with Flat File Support: A Comprehensive Analysis
Table of Contents
In today’s data-driven world, the need for efficient and scalable databases is more critical than ever. Multithreaded embedded databases have gained prominence as they offer the flexibility of embedding a database engine directly into an application, allowing for seamless data storage and retrieval.
These databases are known for their speed, reliability, and versatility. In this article, we will take a deep dive into five popular Multithreaded embedded database with flat file support: SQLite, BoltDB, DBreeze, LevelDB, and RocksDB.
We will compare their features, performance, and use cases to help you make an informed decision when choosing the right database for your project.
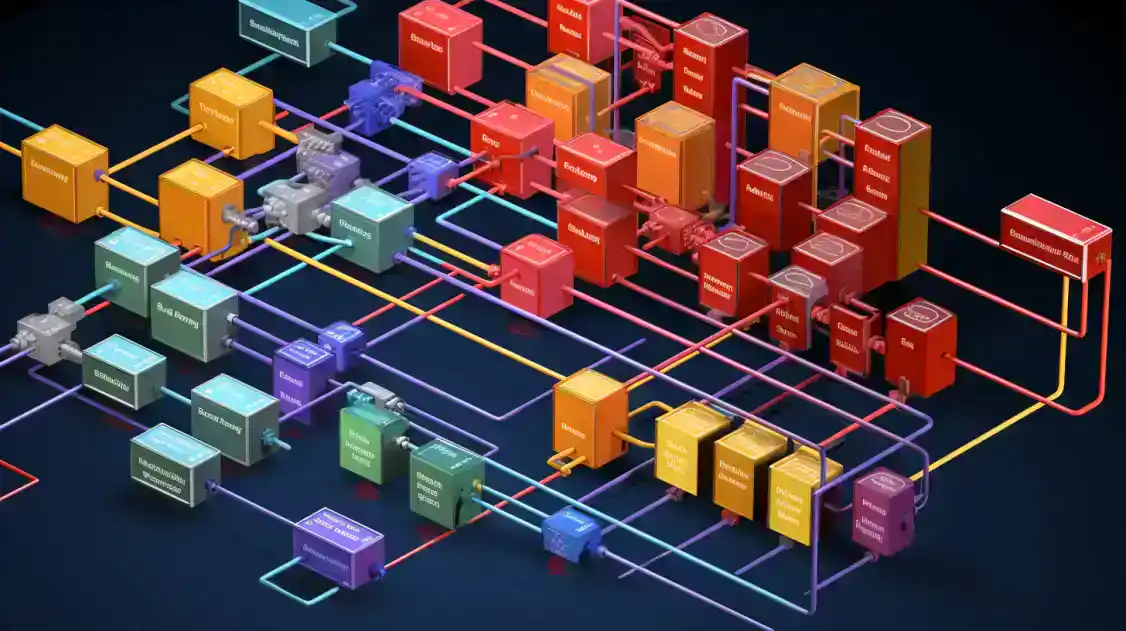
Understanding Multithreaded Embedded Databases
Before we delve into the comparison of the databases, it’s essential to understand what multithreaded embedded databases are and why they are valuable in today’s software development landscape.
Multithreaded embedded databases are database systems designed to be embedded directly into an application, eliminating the need for a separate database server. This approach provides several advantages:
- Simplicity: Embedding databases are easy to integrate into an application since they are just libraries or modules that developers can include in their code.
- Performance: By eliminating network communication and leveraging multi-threading capabilities, embedding databases can offer significantly faster data access compared to traditional client-server databases.
- Portability: Embedding databases are typically lightweight and require minimal setup, making them suitable for use in various environments and platforms.
- Data Isolation: Each application can have its database, ensuring data isolation and reducing the risk of data leakage.
Now that we have a clear understanding of multithreaded embedded databases, let’s explore the five databases in question.
1. SQLite
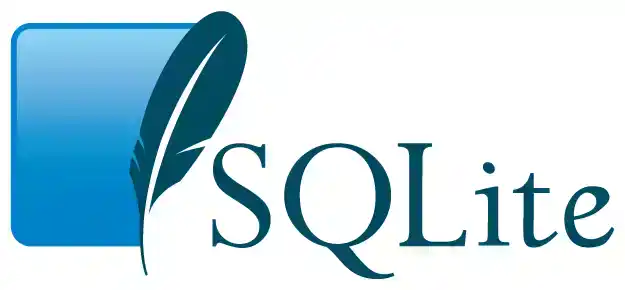
SQLite is a well-known open-source embedded database engine. It is a self-contained, serverless, and zero-configuration database engine, making it a popular choice for small to medium-sized applications.
Key Features:
- ACID Compliance: SQLite is fully ACID compliant, ensuring data integrity and reliability.
- Zero Configuration: No need for a separate server process or configuration files. SQLite databases are just regular files.
- Cross-Platform: SQLite is available on various platforms, including Windows, macOS, Linux, and mobile devices.
- Transaction Support: It supports both single-threaded and multi-threaded access to the database with built-in transaction support.
Use Cases:
SQLite is suitable for small to medium-sized applications that need an embedded database with ACID compliance. It is often used in mobile apps, desktop software, and embedded systems.
Example:
Consider a mobile application that needs to store user data locally. SQLite can be used to create and manage a local database to store user profiles, settings, and application data efficiently.
import sqlite3
# Connect to the SQLite database
conn = sqlite3.connect('my_app.db')
# Create a cursor
cursor = conn.cursor()
# Create a table to store user data
cursor.execute('''CREATE TABLE users
(id INTEGER PRIMARY KEY, name TEXT, email TEXT)''')
# Insert data into the table
cursor.execute("INSERT INTO users (name, email) VALUES (?, ?)", ('John Doe', 'john.doe@example.com'))
# Commit the transaction
conn.commit()
# Query the data
cursor.execute("SELECT * FROM users")
for row in cursor.fetchall():
print(row)
# Close the connection
conn.close()
2. BoltDB
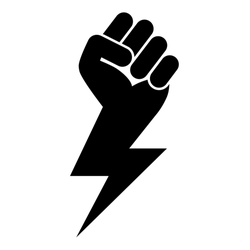
BoltDB is an embedded NoSQL key-value store written in Go. It is designed for high performance and efficiency, making it a popular choice for Go developers.
Key Features:
- Transactions: BoltDB provides full ACID transactions, ensuring data consistency.
- B+Tree Storage Engine: It uses a B+Tree data structure for efficient key-value storage.
- Simple API: BoltDB offers a straightforward API for CRUD (Create, Read, Update, Delete) operations.
- Embeddable: Being written in Go, BoltDB is easy to embed in Go applications.
Use Cases:
BoltDB is an excellent choice for Go applications that require fast, embedded key-value storage. It is commonly used in web servers, microservices, and other Go-based projects.
Example:
Imagine a Go application that needs to cache frequently accessed data. BoltDB can be used to create an efficient in-memory cache.
package main
import (
"log"
"github.com/boltdb/bolt"
)
func main() {
// Open a BoltDB database file
db, err := bolt.Open("my_cache.db", 0600, nil)
if err != nil {
log.Fatal(err)
}
defer db.Close()
// Create or retrieve a bucket
err = db.Update(func(tx *bolt.Tx) error {
bucket, err := tx.CreateBucketIfNotExists([]byte("my_cache"))
if err != nil {
return err
}
// Store key-value pair
err = bucket.Put([]byte("key1"), []byte("value1"))
if err != nil {
return err
}
return nil
})
if err != nil {
log.Fatal(err)
}
// Retrieve a value
err = db.View(func(tx *bolt.Tx) error {
bucket := tx.Bucket([]byte("my_cache"))
if bucket == nil {
return nil
}
value := bucket.Get([]byte("key1"))
log.Printf("Value: %s\n", value)
return nil
})
if err != nil {
log.Fatal(err)
}
}
3. DBreeze
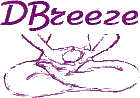
DBreeze is an open-source embedded database engine written in C#. It is designed for high performance and low memory consumption, making it suitable for resource-constrained environments.
Key Features:
- High-Performance Indexing: DBreeze uses a custom indexing algorithm for fast data retrieval.
- Compression: It supports data compression to reduce storage space requirements.
- Multi-Version Concurrency Control (MVCC): DBreeze allows for concurrent data access while maintaining consistency.
- Cross-Platform: While primarily designed for Windows, DBreeze can run on Linux and macOS using Mono.
Use Cases:
DBreeze is an excellent choice for C# applications that require embedded database functionality with a focus on performance and resource efficiency. It can be used in desktop applications, games, and IoT devices.
Example:
Consider a desktop application written in C# that needs to store and retrieve user preferences. DBreeze can be used to create a lightweight embedded database for this purpose.
using DBreeze;
using DBreeze.DataTypes;
class Program
{
static void Main(string[] args)
{
using (var engine = new DBreezeEngine("my_app.db"))
{
using (var transaction = engine.GetTransaction())
{
transaction.SynchronizeTables("user_preferences");
// Store user preferences
transaction.Insert<int, string>("user_preferences", 1, "theme", "dark");
transaction.Insert<int, string>("user_preferences", 1, "language", "english");
// Commit the transaction
transaction.Commit();
}
using (var transaction = engine.GetTransaction())
{
transaction.SynchronizeTables("user_preferences");
// Retrieve user preferences
var theme = transaction.Select<string>("user_preferences", 1, "theme");
var language = transaction.Select<string>("user_preferences", 1, "language");
Console.WriteLine($"Theme: {theme}");
Console.WriteLine($"Language: {language}");
}
}
}
}
4. LevelDB
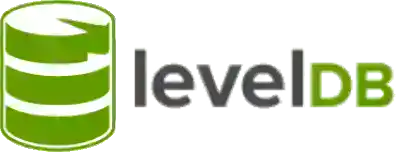
LevelDB is an open-source key-value store developed by Google. It is known for its high performance and simplicity, making it a popular choice for various applications.
Key Features:
- Efficient Key-Value Storage: LevelDB uses a log-structured merge-tree (LSM-tree) for efficient storage and retrieval of key-value pairs.
- Bloom Filters: It employs Bloom filters to reduce disk reads, improving query performance.
- C++ Implementation: LevelDB is implemented in C++, and it provides bindings for various programming languages.
- Concurrency: LevelDB supports concurrent read and write operations.
Use Cases:
LevelDB is suitable for a wide range of applications, from embedded systems to web applications. It is often used in projects where high-performance key-value storage is required.
Example:
Imagine a Python application that needs to store and retrieve user session data efficiently. LevelDB can be used for this purpose.
import leveldb
# Open a LevelDB database
db = leveldb.LevelDB('user_sessions')
# Store user session data
user_id = b'123'
session_data = b'Some session data here'
db.Put(user_id, session_data)
# Retrieve user session data
retrieved_data = db.Get(user_id)
print(retrieved_data.decode('utf-8'))
5. RocksDB
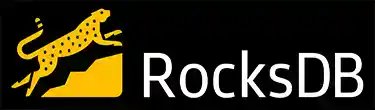
RocksDB is an open-source key-value store developed by Facebook. It is built on LevelDB and is optimized for high write throughput and low latency.
Key Features:
- High Write Throughput: RocksDB is designed for workloads with a high volume of write operations.
- Tiered Storage: It supports tiered storage, allowing you to use both memory and disk storage efficiently.
- Column Families: RocksDB allows data to be organized into column families, providing flexibility in data management.
- Compression: It offers various compression options to reduce storage space requirements.
Use Cases:
RocksDB is an excellent choice for applications that require high write throughput and low-latency access to data. It is commonly used in log storage, time series databases, and distributed systems.
Example:
Consider a Java application that needs to store sensor data from IoT devices. RocksDB can be used to efficiently store and query the sensor data.
import org.rocksdb.*;
public class SensorDataStorage {
public static void main(String[] args) {
try (final Options options = new Options().setCreateIfMissing(true);
final RocksDB db = RocksDB.open(options, "sensor_data")) {
// Store sensor data
byte[] key = "sensor_001".getBytes();
byte[] value = "Temperature: 25°C, Humidity: 60%".getBytes();
db.put(key, value);
// Retrieve sensor data
byte[] retrievedValue = db.get(key);
System.out.println(new String(retrievedValue));
} catch (RocksDBException e) {
System.err.println("Error: " + e.getMessage());
}
}
}
Comparing the Five Databases
To aid you in making an informed decision, let’s conduct a comparative analysis of SQLite, BoltDB, DBreeze, LevelDB, and RocksDB based on various criteria:
Criteria | SQLite | BoltDB | DBreeze | LevelDB | RocksDB |
---|---|---|---|---|---|
Programming Language | C, C++, Python, Java, and more | Go | C# | C++ | C++ |
ACID Compliance | Yes | Yes | Yes | No | No |
Multi-Version Concurrency Control | No | No | Yes | No | No |
Performance | Moderate | High | High | Moderate to High | High |
Disk Space Efficiency | Moderate | Moderate | High | Moderate | Moderate to High |
Compression | No | No | Customizable | No | No |
Cross-Platform Support | Yes | Yes | Yes (via Mono or .NET Core) | Yes | Yes |
Write Throughput | Moderate | High | Good | High | High |
Log-Structured Storage | No | No | No | Yes | Yes |
Automatic Compaction | No | No | No | Yes | Yes |
Column Families (or Similar) | No | No | No | No | Yes |
Tiered Storage | No | No | No | No | Yes |
Integration with Other DBs | No | No | No | No | Apache Cassandra, Hadoop |
GitHub Repository | SQLite | BoltDB | DBreeze | LevelDB | RocksDB |
GitHub Stars | |||||
GitHub Forks | |||||
GitHub Last Commit | |||||
GitHub License | |||||
GitHub Top Language | |||||
GitHub Languages Count | |||||
GitHub Contributors | |||||
GitHub Issues | |||||
GitHub Watchers |
Conclusion
In conclusion, the choice of a multithreaded embedded database with flat file support depends on your specific project requirements. Each of the databases we explored—SQLite, BoltDB, DBreeze, LevelDB, and RocksDB—has its strengths and use cases.
- SQLite is a solid choice for small to medium-sized applications that require an embedded database with ACID compliance and simplicity.
- BoltDB is an excellent option for Go applications that need fast and efficient key-value storage with ACID transactions.
- DBreeze is well-suited for C# applications that demand high performance and low memory consumption.
- LevelDB is versatile and can be used in a wide range of applications, thanks to its efficient key-value storage.
- RocksDB excels in high write throughput scenarios and is ideal for applications with demanding write-intensive workloads.
When choosing a database for your project, consider factors such as the size and complexity of your dataset, read and write performance requirements, and the programming languages you are comfortable with. By carefully evaluating these factors, you can make an informed decision and select the best multithreaded embedded databases to meet your application’s needs.
Featured Insights
The AI Assistant Showdown 2024: Are Flirty, All-Knowing Companions the Future of Silicon Valley?
IBM Watson and Tech Mahindra Propel Sustainable AI
How AI Revolutionizes Hiring and Retention for SMBs
Strava Supercharges Training with AI, Boosts Fairness for Athletes (Especially Women!)
YouTube Training Data and AI Video Models: Did Google Reveal OpenAI’s Breakthrough?
Is Slack AI Data Privacy Sacrificing Your Privacy for Convenience?