Understanding Data Structures: Essential Need, Types & Classification in 2024
Table of Contents
What is a Data Structure?
Data structures are essential concepts in computer science and programming. They are specific methods of organizing and storing data in a computer to enable efficient access and modification. Data structures are crucial for managing extensive amounts of data in applications like databases, internet indexing services, and large-scale simulations.
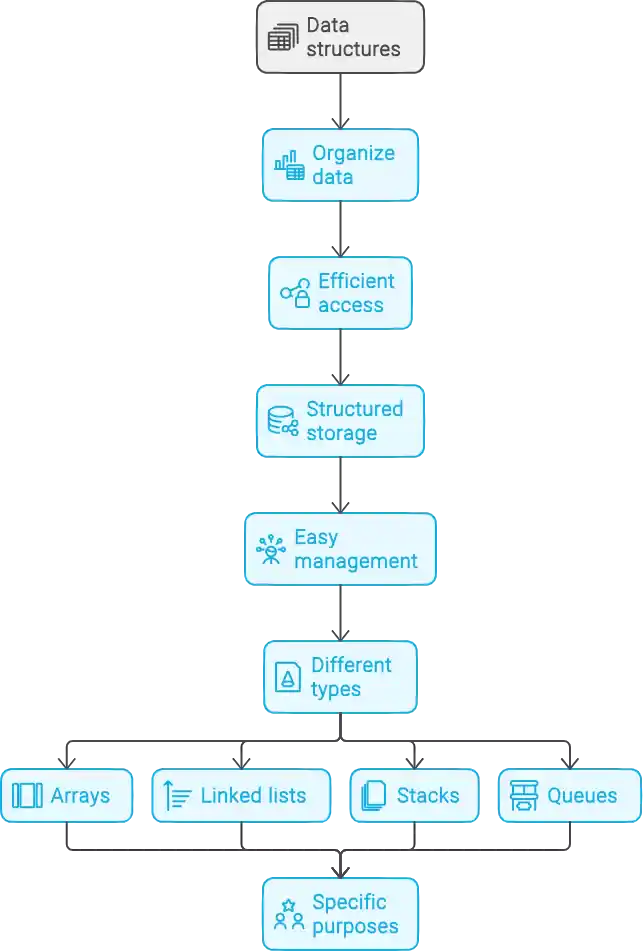
Understanding data structure is crucial for designing efficient algorithms and writing high-performance software. In simple terms, a data structure can be thought of as a container that stores data in a specific layout. This layout allows for data to be easily accessed, modified, and utilized for various operations.
Need for Data Structures
The need for data structure arises from the necessity to handle data efficiently. With the explosion of data in recent years, efficient data handling is more important than ever. Whether you are developing a small application or a large-scale system, choosing the right data structure can significantly impact performance and resource utilization.
They help in organizing data so that operations like searching, insertion, deletion, and sorting can be performed efficiently. They are essential in solving complex computational problems where performance is a critical concern. With appropriate data structure, the software can become fast, consume excessive resources, and meet user expectations.
Types of Data Structures
There are various types of data structures, each suited to different kinds of applications. The primary types include arrays, linked lists, stacks, queues, trees, and graphs. Understanding these basic types and their use cases is essential for any programmer or computer scientist.
Arrays
Arrays are a basic and commonly used type of data structure. An array is a collection of elements, each identified by an index or a key. The main advantage of arrays is their simplicity and the ability to access elements quickly using an index.
However, arrays have a fixed size, and inserting or deleting elements can be costly since it may require shifting other elements. Despite these limitations, arrays are incredibly efficient for scenarios where the size of the data set is known in advance and frequent insertions and deletions are not required.
Linked Lists
Linked lists are flexible data structures made up of nodes. Each node holds data and a pointer to the next node. They are beneficial because they enable quick insertion and removal of elements without having to move other elements around.
However, linked lists do not provide constant-time access to elements like arrays. To access an element, one must traverse the list from the beginning to the desired position, which can be time-consuming for large lists.
Stacks
Stacks are structures that follow the Last In, First Out (LIFO) principle. Elements are added and removed from the top. They are used when the most recent element needs to be accessed first, like in function calls, expression evaluation, and backtracking algorithms.
Queues
Queues are straight data structures that follow the FIFO principle. Items are inserted at the back and taken out from the front. They are used in situations where items must be handled in the same order they were added, like in task scheduling, buffering, and breadth-first search algorithms.
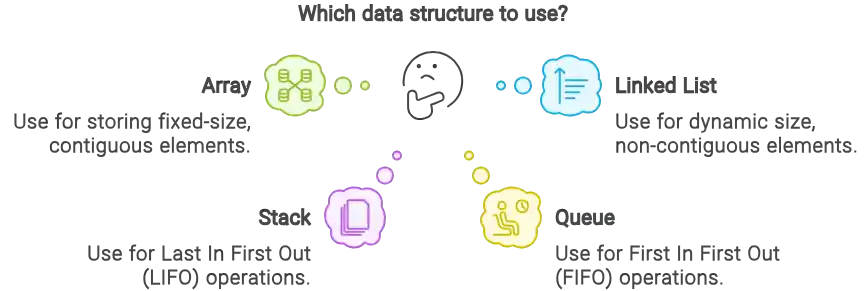
Trees
Trees are structures made up of nodes connected by edges. Each tree has a root node and zero or more child nodes. Trees are used to represent hierarchical relationships, such as organizational structures, file systems, and XML data.
Binary trees, where each node has at most two children, are a common type of tree. Trees allow for efficient searching, insertion, and deletion operations and are used in many algorithms and applications.
Graphs
Graphs are complex data structures that consist of a set of nodes (vertices) connected by edges. Graphs can be used to represent various real-world problems, such as social networks, transportation networks, and communication networks.
Graphs can have two types: directed and undirected. In a directed graph, edges show a one-way relationship. In an undirected graph, edges show a two-way relationship. Graph algorithms help in finding the shortest path, detecting cycles, and navigating networks.
Classification of Data Structures
They can be broadly classified into two categories: linear and non-linear data structures. This classification is based on how the data elements are arranged and interconnected.
Linear Data Structures
Linear data structure arranges data elements in a sequential manner, where each element is connected to its previous and next elements. Examples of linear data structures include arrays, linked lists, stacks, and queues. These structures are easy to implement, making them suitable for many applications.
Linear structures are used when data needs to be processed in a specific order. They provide simple and efficient mechanisms for accessing and managing data, which is why they are widely used in various programming scenarios.
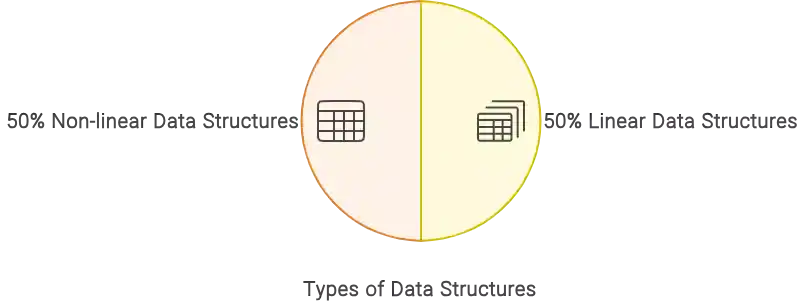
Non-Linear Data Structure
Non-linear data structure arranges data elements in a hierarchical or interconnected manner, where elements are not arranged in a sequential order. Examples of non-linear structures include trees and graphs. These structures are more complex but offer powerful capabilities for representing relationships between data elements.
Non-linear structures are used in applications where hierarchical relationships or network connections need to be represented. They provide efficient mechanisms for complex data processing tasks, such as searching, sorting, and traversal.
Where Do We See Data Structures in Action?
They are the hidden heroes behind countless applications in our daily lives:
- Social Media: Data structures manage user profiles, connections, and news feeds efficiently.
- Search Engines: They leverage data structures like hash tables to store and retrieve vast amounts of web pages and their content for lightning-fast search results.
- Operating Systems: Data structures manage files, folders, and memory allocation, ensuring smooth system operation.
- Gaming: Game development heavily relies on data structures to represent game worlds, characters, and interactions, creating immersive experiences.
- Machine Learning: Data structures are instrumental in organizing and processing large datasets used to train and run machine learning algorithms.
The list goes on! Data structure is the fundamental building block for a wide range of software applications, silently ensuring their efficiency and functionality.
Popular Data Structures and Their Uses
Different types of problems require different data structures. Here are a few commonly used data structures and their purposes:
Hash Tables
– Implementation: Used to implement associative arrays or mappings of key-value pairs.
– Efficiency: Provide efficient insertion, deletion, and lookup operations.
Applications:
– Database Indexing: Quickly retrieve data using keys.
– Caching: Store and access frequently used data efficiently.
– Sets and Dictionaries: Implement sets and dictionaries for fast data retrieval.
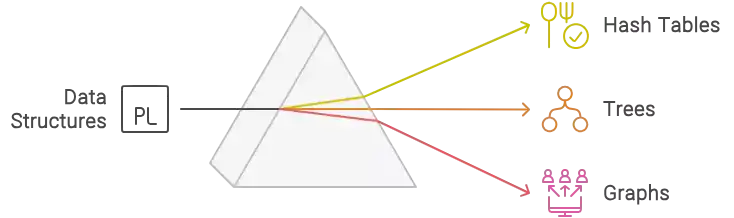
Data Structures for Programming
Algorithms: Essential for implementing various algorithms and solving computational problems.
Trees and Graphs:
– Search Algorithms: Used in algorithms like binary search trees and graph traversal algorithms (e.g., depth-first search, breadth-first search).
Stacks and Queues:
– Parsing: Utilized in syntax parsing for compilers and interpreters.
– Scheduling: Employed in task scheduling and resource management algorithms.
Performance Impact: Choosing the right data structure can significantly enhance the performance and efficiency of a program.
Conclusion
Data structures are essential components in the field of computer science and programming. They provide efficient ways to organize, store, and manipulate data, enabling the development of high-performance software. Understanding the need for data structure, their types, and their applications is essential for any programmer or computer scientist.
In 2024, as data continues to grow exponentially, the importance of efficient data structure and algorithms cannot be overstated. Whether you are developing a small application or a large-scale system, mastering data structure will help you design efficient solutions and tackle complex computational problems.
FAQs
1. What are the most common data structures?
Arrays, linked lists, stacks, queues, trees, and graphs are some of the most widely used data structures.
2. How do I choose the right data structure for my program?
The best data structure for your program depends on the specific problem you’re trying to solve. Consider factors like the type of data you’re working with, the operations you need to perform on it (e.g., insertion, deletion, search), and the desired efficiency.
3. Where can I learn more about data structure?
There are numerous online resources, tutorials, and courses available to help you learn more about data structure. Many programming languages also provide built-in implementations of common data structures.
4. Are data structures important for web development?
Absolutely! Data structures are crucial for web development tasks like managing user data, storing website content, and handling network requests efficiently.
5. How can data structures improve my programming skills?
A solid understanding of data structure will enable you to write cleaner, more efficient, and maintainable code. It will also empower you to design better algorithms and solve problems more effectively.
Featured Insights
Integrating Real-Time Weather Data into Your Travel and Navigation App
The Future is Now: AI and ML Technologies Reshaping Healthcare
Introduction to Digital Twin Technology in Manufacturing with Unprecedented Efficiency and Innovation
Adobe’s Firefly AI: New Tools for Photoshop and Illustrator
Introducing Meta Imagine Me: Your Digital Doppelganger
AI in Gaming is Transforming the Industry