Table of Contents
The React ecosystem has always been dynamic, with regular updates bringing significant improvements for developers. The previous version introduced several groundbreaking features, but the buzz around the latest release indicates even more exciting advancements.
In this blog, we’ll explore the differences between the two versions, comparing their features, performance enhancements, and how these changes affect developers.
A Quick Recap of React 18
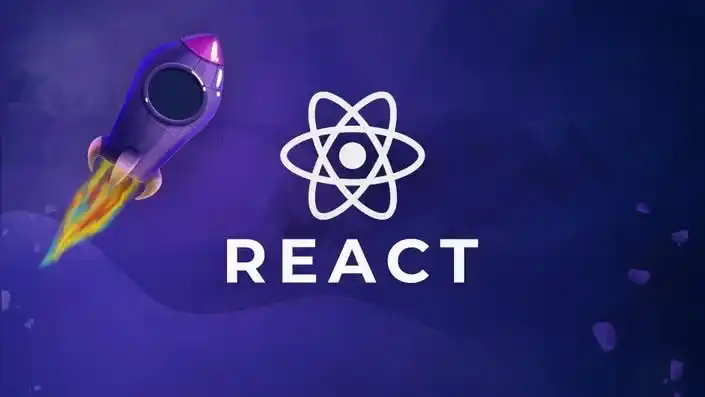
The earlier release was a major update, introducing features that revolutionized the way developers handle rendering and state management. Some of the key highlights were:
1. Concurrent Rendering
The previous version introduced concurrent rendering, enabling the library to interrupt and pause rendering processes to prioritize more critical updates. This feature improved the responsiveness of applications, especially in cases where heavy computations were involved.
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
The createRoot
API was pivotal in enabling concurrent rendering by default.
2. Automatic Batching
Automatic batching streamlined state updates within event handlers and async functions. Previously, developers had to wrap state updates manually using unstable_batchedUpdates
to achieve similar behavior.
function handleClick() {
setState1(newValue1);
setState2(newValue2);
// Both updates are batched automatically.
}
3. Transition API
The Transition API allowed developers to distinguish between urgent and non-urgent updates, enhancing the user experience by prioritizing critical tasks.
import { startTransition } from 'react';
startTransition(() => {
setNonUrgentState(newValue);
});
4. Suspense Improvements
Suspense became more robust in the earlier release, allowing developers to handle loading states for asynchronous data fetching seamlessly.
5. Server-Side Rendering (SSR) Enhancements
The streaming
capability in the previous release improved the performance of server-rendered apps, offering faster load times and better SEO.
6. Strict Mode Enhancements
The earlier version’s Strict Mode added development-only checks for detecting unexpected side effects in applications, ensuring better debugging and long-term maintainability.
<React.StrictMode>
<App />
</React.StrictMode>
Strict Mode is invaluable for catching issues during development.
Enter React 19: What’s New?
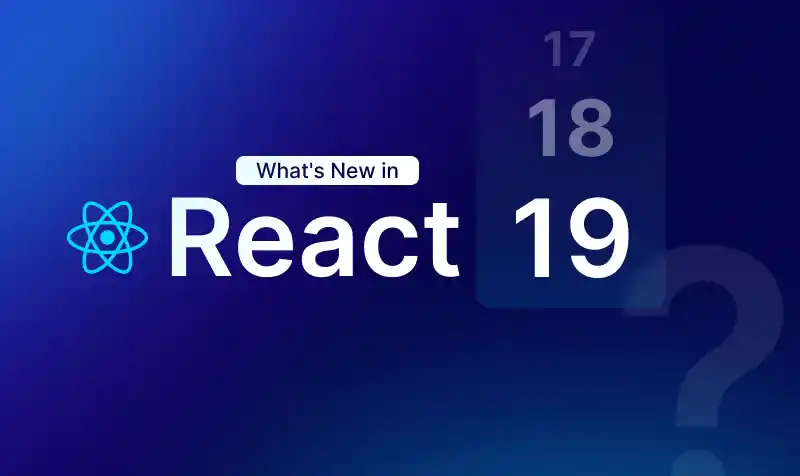
The latest version builds upon its predecessor’s foundation, focusing on developer experience, performance, and feature extensibility. Here are the key updates:
1. Optimized Concurrent Rendering
While the earlier version introduced concurrent rendering, the new release refines it further. It introduces an improved scheduling algorithm that dynamically adapts to user interactions, making apps feel even more fluid.
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />, { adaptiveScheduling: true });
This feature reduces unnecessary re-renders and improves rendering efficiency.
2. Enhanced Server Components
The latest version expands the functionality of Server Components, enabling developers to fetch and stream data on the server more efficiently. It seamlessly integrates with frameworks such as Next.js.
// App.server.js
export default function App({ data }) {
return <div>{data}</div>;
}
This update minimizes the need for client-side data fetching, reducing bundle sizes and improving performance.
3. Improved Error Handling
The new release enhances error boundaries with more granular control and better debugging tools. Developers can now handle specific errors more effectively.
function ErrorBoundary({ children }) {
try {
return children;
} catch (error) {
return <FallbackComponent error={error} />;
}
}
Error handling is now more intuitive, giving developers finer control over how errors are managed.
4. Suspense for Data Fetching
The latest version extends Suspense’s capabilities, making it easier to handle asynchronous operations. This update works seamlessly with React’s new useData
hook.
const data = useData(fetchData);
return <Suspense fallback={<LoadingSpinner />}>{data}</Suspense>;
Suspense’s integration with hooks like useData
simplifies asynchronous workflows, reducing boilerplate code.
5. Improved Developer Tooling
The React Developer Tools extension gets a significant upgrade, offering deeper insights into rendering performance and state management. The new profiler features allow developers to pinpoint performance bottlenecks with ease.
6. Cross-Browser Compatibility Improvements
The latest version includes enhanced polyfills, ensuring a consistent experience across older browsers without additional configuration. This makes it a more accessible choice for legacy projects.
Performance Benchmarks
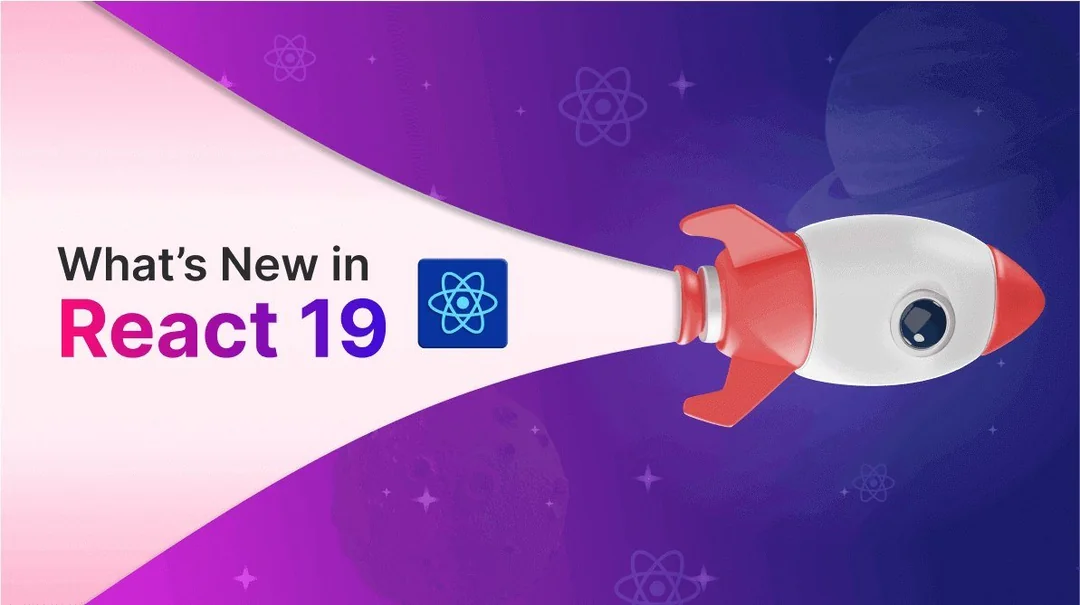
Rendering Speed
The latest release shows marked improvements in rendering speed, particularly for apps with high user interaction. Benchmarks reveal a 20% improvement in rendering large lists compared to its predecessor.
Memory Efficiency
Memory usage has been optimized in the new version, reducing the overhead caused by large component trees. This translates to smoother performance on resource-constrained devices.
Network Performance
With enhanced server-side rendering and streaming capabilities, the latest version reduces initial load times for server-rendered applications, providing a better user experience.
Migration from React 18 to React 19
The new release’s backward compatibility ensures a smooth transition for developers upgrading from the earlier version. Key migration steps include:
- Upgrade React and React DOM:
npm install react@19 react-dom@19
- Review Deprecated APIs: The new release deprecates some lesser-used APIs, which will require code updates. Check the official migration guide for more information.
- Test Concurrent Features: Ensure existing concurrent features align with the new scheduling algorithms.
- Update Build Tools: If you’re using a framework like Next.js or Vite, ensure you’re on the latest versions that support the new release.
Feature Comparison
Feature | React 18 | React 19 |
---|---|---|
Concurrent Rendering | Basic | Adaptive Scheduling |
Server Components | Experimental | Fully Supported |
Suspense | Improved | Enhanced for Data Fetching |
Error Handling | Basic | Granular and Debuggable |
Developer Tooling | Standard | Advanced Insights |
Cross-Browser Support | Standard | Improved |
Use Cases and Real-World Applications
When to Stick with React 18
- Existing projects that rely on stable features without immediate need for the enhancements in the latest release.
- Teams prioritizing stability over the adoption of cutting-edge updates.
When to Upgrade to React 19
- Applications with high user interaction demanding improved responsiveness.
- Projects utilizing Server Components for better performance.
- Developers seeking advanced tooling and debugging capabilities.
- Teams focused on delivering optimized experiences for legacy browser users.
Conclusion
The earlier release laid a robust foundation with concurrent rendering, automatic batching, and enhanced Suspense. The latest release builds upon these innovations, introducing refined rendering, better server-side capabilities, advanced tooling, and improved compatibility. Whether you’re building a new app or maintaining an existing one, the new release offers compelling reasons to upgrade, especially for performance-critical applications.
As the library continues to evolve, developers can look forward to building faster, more efficient, and user-friendly applications. Which version are you using or planning to use for your next project? Let us know in the comments!