Table of Contents
Object-Oriented Programming: An Introduction
The world of programming is made up of various programming concepts and patterns. Different software developers use different patterns when programming. One of the most well-known and widely used concepts is object-oriented programming (OOP). It’s not just a programming paradigm; it’s a philosophy.
At its core, OOP represents complex software systems as interactive objects, reflecting the real world in which objects interact to perform tasks. This programming approach not only improves code reuse and maintainability but also allows developers to think more abstractly about their solutions.
This blog post will cover the history, concepts, principles, benefits, languages, and alternatives to OOP. So without further ado, let us get started.
A Brief History of OOP (Object-Oriented Programming)
The seeds of OOP were sown in the 1960s with the creation of Simula, a language designed specifically for simulating real-world systems.
However, the true origins of OOP are often attributed to Alan Kay and his team at Xerox PARC in the 1970s, who created Smalltalk, a language that fully embraced the object-oriented approach.
Since then, OOP principles have been incorporated into many programming languages, influencing how we design, develop, and support software applications.
The Structure of OOP
The structure of OOP is centered around classes and objects, where classes serve as templates for creating objects and encapsulating data and behaviors. This organization allows for a modular design, promoting code reusability and simplification of complex software systems.
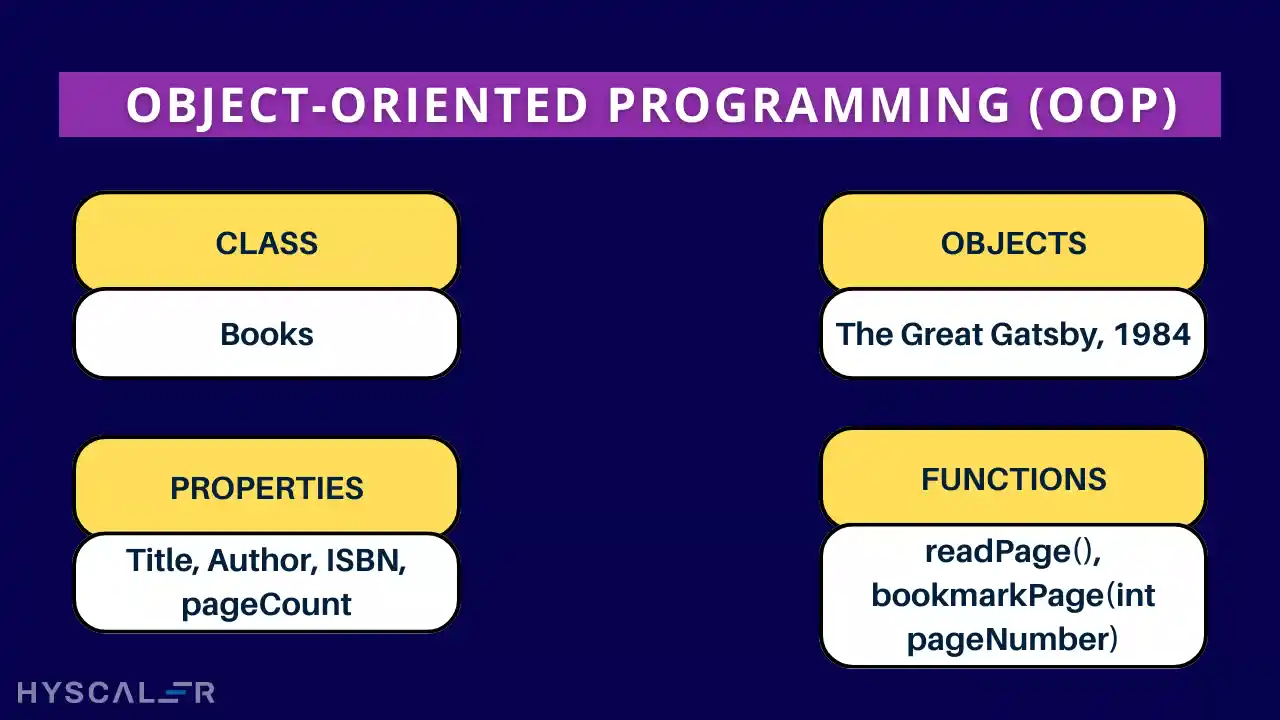
Objects
Objects are the primary building blocks of Object-oriented programming. They are self-contained pieces of code that include properties and methods. An object is a class instance that represents one specific instance of something within a program. For example, if you were developing a library management program, each book could be an object with properties like title, author, and publication date, as well as methods like check out, return, and renew.
Classes
Classes serve as blueprints for objects. They specify the properties and methods that each object in the class will have. In the library example, a class could be ‘Book’, with properties like ‘title’, ‘author’, and ‘publication date’, and methods like ‘check out’, ‘return’, and’renew’. When an object is created, or ‘instantiated’ by a class, it inherits the properties and methods.
Inheritance
Inheritance is an important feature of OOP, allowing classes to inherit properties and methods from other classes. This is useful for deriving more specific subclasses from general classes, reducing redundancy, and increasing reusability. In the library example, you might have a general ‘Book’ class, and then more specific subclasses like ‘FictionBook’ or ‘NonFictionBook’ that inherit the ‘Book’ class’s properties and methods while also having some of their own.
The Principles of OOP
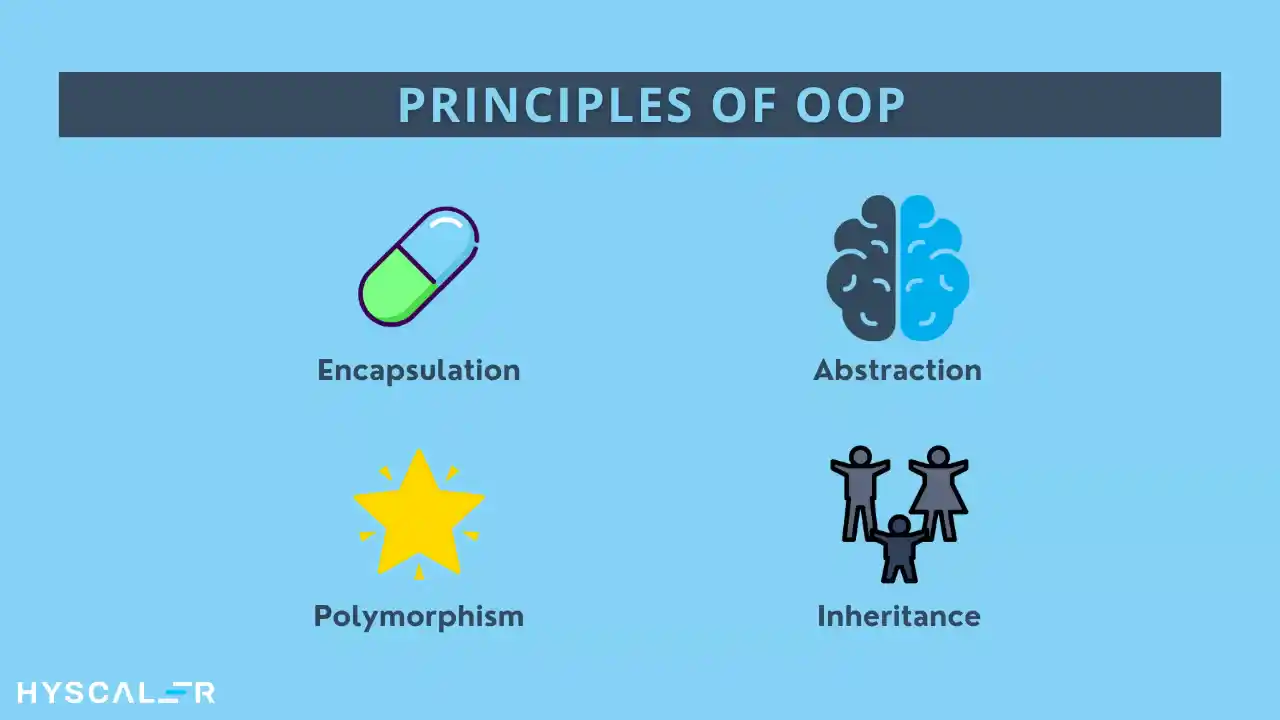
Encapsulation, abstraction, polymorphism, and inheritance are the four fundamental concepts of object-oriented programming. These principles guide the structure and organization of OOP programming, resulting in code that is efficient, readable, and reusable.
Encapsulation
Encapsulation is the principle of enclosing data and operations within a class. This technique enables data concealing since the data of one class is hidden from other classes. It can only be accessible via the methods of the current class. Thus, it is also known as data concealing. This idea improves security and reduces data corruption.
Abstraction
Abstraction is the notion of simplifying complex systems by modeling problem-specific classes and working at the appropriate level of inheritance for each component of the problem. This entails concealing complex elements and displaying only the important characteristics. Abstraction enables the programmer to construct a broad idea or concept that can be easily understood and utilized as the foundation for more complicated code.
Polymorphism
Polymorphism is the concept that an object can take on several forms. In other words, a single interface can be used to represent a diverse range of actions. This approach allows methods to behave differently depending on the object that invokes them. This adds flexibility and simplicity to the code.
Inheritance
Inheritance is the principle that allows one class to inherit traits and attributes from another. This technique promotes code reuse by allowing child classes to inherit properties and methods from their parent classes. It also enables the design of more particular classes using basic classes as a base.
A thorough comprehension of these principles is required for any programmer working with OOP. They give a foundation for creating flexible and reusable code, which improves programming efficiency and management.
The Benefits of Object-Oriented Programming
Object-oriented programming provides several significant advantages, making it an appealing option for many programmers and developers. These advantages arise from OOP’s fundamental ideas and structure, and they can have a substantial impact on both the development process and the final output.
Modularity
In the world of object-oriented programming, each object is a standalone module. Individual objects can be changed without affecting the whole system due to their modularity. This element of Object-Oriented Programming facilitates not only the process of upgrading and improving the system but also the understanding and management of the codebase.
Reusability
Objects and classes in Object-Oriented Programming are intended to be reused throughout an application, or even across multiple apps. This reusability saves time and effort since programmers can use existing classes and objects rather than building new ones from the start. It also increases consistency throughout the application because the same objects and classes are utilized in several situations.
Flexibility
OOP provides a high level of flexibility. Existing classes can be used to construct new objects, making it possible to build large systems from simple building blocks. This flexibility includes the ability to modify and extend existing classes and objects, making it easier to adapt the system to new requirements or changes.
Maintainability
Each object in an Object-Oriented Programming system manages its upkeep. Encapsulating data and methods within distinct objects make it easier to maintain and update the system. It also decreases the likelihood of errors and defects, because modifications to one item are unlikely to affect others.
The advantages of OOP—modularity, reusability, adaptability, and maintainability—make it an effective tool for programmers and developers. Understanding and successfully utilizing these benefits allows one to build durable, efficient, and flexible systems.
The Popular OOP Languages
Object-Oriented Programming has been implemented in various programming languages. Here are some of the most popular ones:
Java
Java is one of the most widely used OOP languages. Developed by Sun Microsystems (now Oracle Corporation), Java is known for its “write once, run anywhere” philosophy, meaning compiled Java code can run on all platforms without needing to be recompiled.
C++
C++ is another popular Programming language, developed by Bjarne Stroustrup at Bell Labs. C++ is a powerful, high-performance language that is commonly used for system/software development and game programming. It allows object-oriented programming, but also procedural programming.
Python
Python is a high-level, interpreted OOP language that has gained popularity for its clear, readable syntax. Python supports multiple programming paradigms, including object-oriented, imperative, functional, and procedural styles. It is often used in data analysis, machine learning, AI, web development, and scripting.
C#
C#, pronounced as “C sharp,” is an OOP language developed by Microsoft. It is a part of the .NET framework and is often used for Windows desktop applications and game development with the Unity game engine.
Objective-C
Objective-C is an OOP language that was used by Apple for the OS X and iOS operating systems, and their APIs. It was superseded by Swift in 2014, but it’s still in use for existing applications and system-level programming. Objective-C extends the C programming language with the addition of Smalltalk-style messaging.
Each of these languages has its strengths and use cases, but all of them utilize the principles of Object-Oriented Programming to allow for clear, modular, and reusable code.
Alternatives to OOP
While OOP is a powerful and widely used paradigm, it’s not the only approach to programming. Other paradigms offer different ways to structure and organize code, and each has its strengths and weaknesses. Here are three alternatives to OOP:
Procedural Programming
Procedural programming is a programming paradigm based on the concept of procedure calls. Procedures, also known as routines or subroutines, are blocks of code that can be invoked from different parts of a program. This paradigm is characterized by a top-down structure, where a program starts at the top and executes each procedure in order, passing data from one procedure to the next.
Functional Programming
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. In functional programming, functions are first-class citizens, meaning they can be passed as arguments to other functions, returned as values from other functions, and assigned to variables. This approach promotes a declarative programming style, where the logic of computation is expressed without describing its control flow.
Logical Programming
Logical programming is a paradigm based on formal logic. A program written in a logical programming language is a set of sentences in logical form, expressing facts and rules about some problem domain. The most commonly known logical programming language is Prolog. In logical programming, you express a problem by defining its facts and rules, and the system solves it by making logical inferences.
Each of these paradigms offers a different approach to problem-solving, and understanding them can broaden a programmer’s toolkit and perspective. It’s important to choose the right tool for the job, and sometimes, that tool might not be OOP.
Conclusion
Object-oriented programming (OOP) is a valuable technique in the toolbox of modern programmers. It enables the building of modular and adaptable code using objects and classes. OOP’s modularity means that each object is a self-contained entity that can be independently modified and tested, which improves application dependability and resilience. Its versatility stems from the ability to generate new objects from existing classes, which promotes code reuse and shortens development time.
To properly master OOP, one must not only comprehend the principles but also be able to apply them in real-world settings. This necessitates hands-on experience and ongoing learning.
In conclusion, OOP is a versatile and strong programming paradigm that provides numerous benefits. Whether you’re a new programmer or an experienced developer trying to improve your skills, understanding and properly using OOP can significantly improve your coding talents.