Table of Contents
1. Introduction to the JavaScript Event Loop
When you hear “Event Loop”, what comes to mind? Maybe a new JavaScript framework? Or something you’d rather not touch with a 10-foot pole? Don’t worry. The Event Loop isn’t some mysterious beast—it’s actually one of the most important concepts in asynchronous programming.
In this guide, we’ll break down the JavaScript Event Loop, explain how it works with the call stack, microtasks, and task queues, and share practical examples to level up your understanding of JavaScript’s non-blocking nature.
Let’s demystify this concept and take your JavaScript skills to the next level!
2. Key Components of the Event Loop
The JavaScript Event Loop interacts with several key components. Here’s a quick overview:
a. Call Stack
The Call Stack is where all synchronous code gets executed. It follows a simple rule: last in, first out (LIFO).
Example:
function greet() {
console.log("Hello!");
}
greet();
- The
greet()
function is pushed onto the stack. console.log("Hello!")
is executed.- The function is popped off the stack.
b. Web APIs
Web APIs handle asynchronous tasks like timers, HTTP requests, or DOM events outside the Call Stack.
Example: When you use setTimeout
, the timer operates in the Web API environment while the main thread continues execution.
c. Task Queue
The Task Queue stores macrotasks, such as setTimeout
callbacks, waiting to be executed after the Call Stack is clear.
d. Microtask Queue
The Microtask Queue holds higher-priority tasks like resolved promises or MutationObserver
callbacks. These run before macrotasks but after the Call Stack clears.
3. How the JavaScript Event Loop Works
Here’s how the Event Loop operates:
a. Synchronous Code Runs First:
The Event Loop starts by running synchronous tasks in the Call Stack.
b. Handle Asynchronous Tasks:
- If an asynchronous task is encountered, it’s offloaded to a Web API.
- Once the task completes, its callback enters the appropriate queue.
c. Microtasks First:
The Event Loop prioritizes tasks from the Microtask Queue before processing the Task Queue.
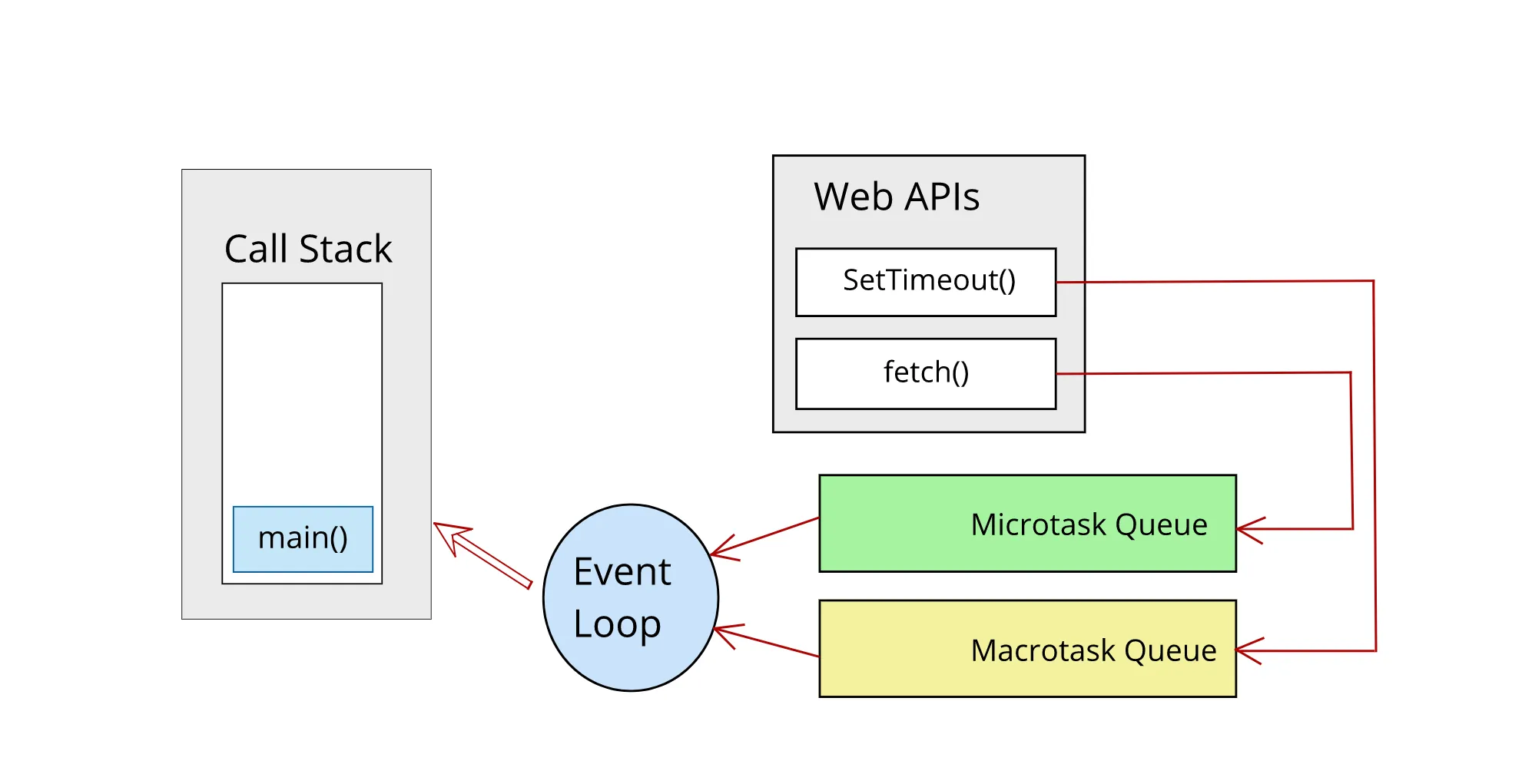
4. Breaking Down Asynchronous Code Examples
Let’s see the Event Loop in action:
Example: setTimeout vs. Promises
console.log("Start");
setTimeout(() => {
console.log("Timeout");
}, 0);
Promise.resolve().then(() => {
console.log("Promise");
});
console.log("End");
Output:
Start
End
Promise
Timeout
console.log("Start")
executes first (synchronous).setTimeout
schedules a callback in the Task Queue.Promise.resolve
schedules a callback in the Microtask Queue.console.log("End")
runs next (synchronous).- The Microtask Queue processes
Promise
first. - Finally, the Task Queue processes
setTimeout
.
Example: Async/Await and the Event Loop
async function fetchData() {
console.log("Fetching...");
const data = await new Promise((resolve) =>
setTimeout(() => resolve("Data Received"), 1000)
);
console.log(data);
}
console.log("Start");
fetchData();
console.log("End");
Output:
Start
Fetching...
End
Data Received
Explanation:
fetchData
logs “Fetching…” and pauses atawait
.- The Event Loop continues with “End”.
- After the promise resolves, “Data Received” is logged.
Common Misconceptions About the Event Loop
- “setTimeout with 0ms runs immediately.”
Not true. It’s queued in the Task Queue and waits for synchronous code and microtasks to complete. - “Promises and setTimeout are the same.”
Promises belong to the Microtask Queue and are executed beforesetTimeout
(macrotasks).
Conclusion
The javaScript Event Loop is the backbone of asynchronous programming. Understanding how it handles tasks, from the Call Stack to the Microtask Queue, unlocks a new level of JavaScript mastery.
Think of the Event Loop as a well-choreographed dance between synchronous and asynchronous code. Once you understand the rhythm, you’ll write cleaner, faster, and more maintainable code.
So the next time you encounter a tricky async bug, ask yourself: What’s the Event Loop doing right now?
Stay curious, keep experimenting, and happy coding!