Table of Contents
In the fast-paced world of web development, creating visually stunning, highly responsive, and consistent user interfaces is a daunting task. For developers and designers, striking the perfect balance between functionality and aesthetics requires a powerful set of tools. React and Tailwind CSS have emerged as a winning combination for building modern design systems that can take your projects from pixels to perfection.
In this comprehensive guide, we’ll dive deep into the process of mastering design systems with React and Tailwind. From understanding the core principles of design systems to leveraging these powerful technologies, you’ll gain the knowledge to elevate your development workflow and deliver exceptional user experiences.
What Is a Design System?
A design system is a collection of reusable components, guidelines, and best practices that work together to create a unified user experience. It provides a shared language for designers and developers, ensuring consistency across all aspects of a digital product.
Key Components of a Design System:
- Foundations: Colors, typography, spacing, and grid systems that form the basic building blocks of your interface.
- Components: Buttons, forms, modals, and other UI elements that can be reused across your application.
- Patterns: Reusable solutions for common design problems and user interactions.
- Guidelines: Comprehensive documentation that outlines best practices, usage instructions, and accessibility standards.
- Design Tokens: Named entities that store visual design attributes for easy maintenance and scaling.
Why React and Tailwind CSS for Design Systems?
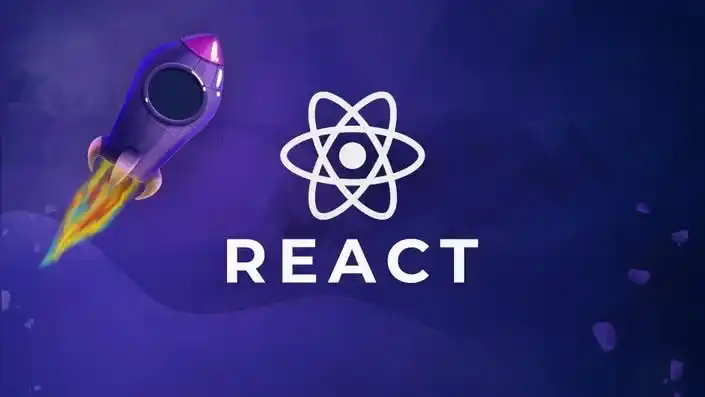
React, a declarative JavaScript library, and Tailwind CSS, a utility-first CSS framework, complement each other beautifully in creating robust design systems. Here’s a detailed look at why this combination works so well:
Component-Based Architecture
React allows you to build encapsulated components that can manage their state. Combined with Tailwind’s utility classes, you can create reusable, customizable components quickly. This approach promotes:
- Clear separation of concerns
- Easy maintenance and updates
- Consistent behavior across different contexts
- Improved development efficiency
Consistency and Scalability
Tailwind’s utility classes promote consistency, while React’s modularity ensures that your design system scales effortlessly as your project grows. Benefits include:
- Standardized spacing and sizing
- Consistent color usage
- Predictable typography
- Maintainable codebase
Rapid Prototyping
With Tailwind’s pre-defined classes and React’s reusable components, you can go from design to deployment at lightning speed:
- Quick iteration on designs
- Immediate visual feedback
- Reduced time-to-market
- Efficient development cycle
Customization and Flexibility
Both tools offer high levels of customization. Tailwind’s configuration file lets you extend or override default styles, while React’s props and state management provide endless possibilities for component behavior.
Building the Foundation
Before diving into code, let’s establish the foundation of our design system. This includes:
1. Defining Your Brand Identity
Every design system starts with a brand identity. Define your color palette, typography, and spacing guidelines.
Example Configuration:
// tailwind.config.js
module.exports = {
theme: {
extend: {
colors: {
primary: '#1E40AF',
secondary: '#1E293B',
accent: '#F59E0B',
},
fontFamily: {
sans: ['Inter', 'sans-serif'],
serif: ['Merriweather', 'serif'],
},
},
},
};
Typography System
Define a clear typography hierarchy:
- Font families for different purposes
- Font sizes and line heights
- Font weights
- Letter spacing
2. Setting Up Advanced Component Architecture
Create a structured approach to component development:
Atomic Design Methodology
Organize components following atomic design principles:
- Atoms (basic building blocks)
- Molecules (simple component combinations)
- Organisms (complex component combinations)
- Templates (page-level layouts)
- Pages (specific instances of templates)
Component Example: Input Field System
Input = ({
label,
error,
helper,
icon,
variant = 'default',
size = 'medium',
...props
}) => {
const variants = {
default: 'border-gray-300 focus:border-primary-500',
error: 'border-error-500 focus:border-error-600',
success: 'border-success-500 focus:border-success-600',
};
const sizes = {
small: 'px-2 py-1 text-sm',
medium: 'px-3 py-2',
large: 'px-4 py-3 text-lg',
};
return (
<div className="flex flex-col gap-1">
{label && (
<label className="text-sm font-medium text-gray-700">
{label}
</label>
)}
<div className="relative">
{icon && (
<div className="absolute inset-y-0 left-0 pl-3 flex items-center">
{icon}
</div>
)}
<input
className={`
w-full rounded-md border
${variants[variant]}
${sizes[size]}
${icon ? 'pl-10' : ''}
transition-colors duration-200
focus:ring-2 focus:ring-offset-2 focus:outline-none
`}
{...props}
/>
</div>
{helper && (
<p className={`text-sm ${error ? 'text-error-500' : 'text-gray-500'}`}>
{helper}
</p>
)}
</div>
);
};
3. Setting Up Tailwind CSS
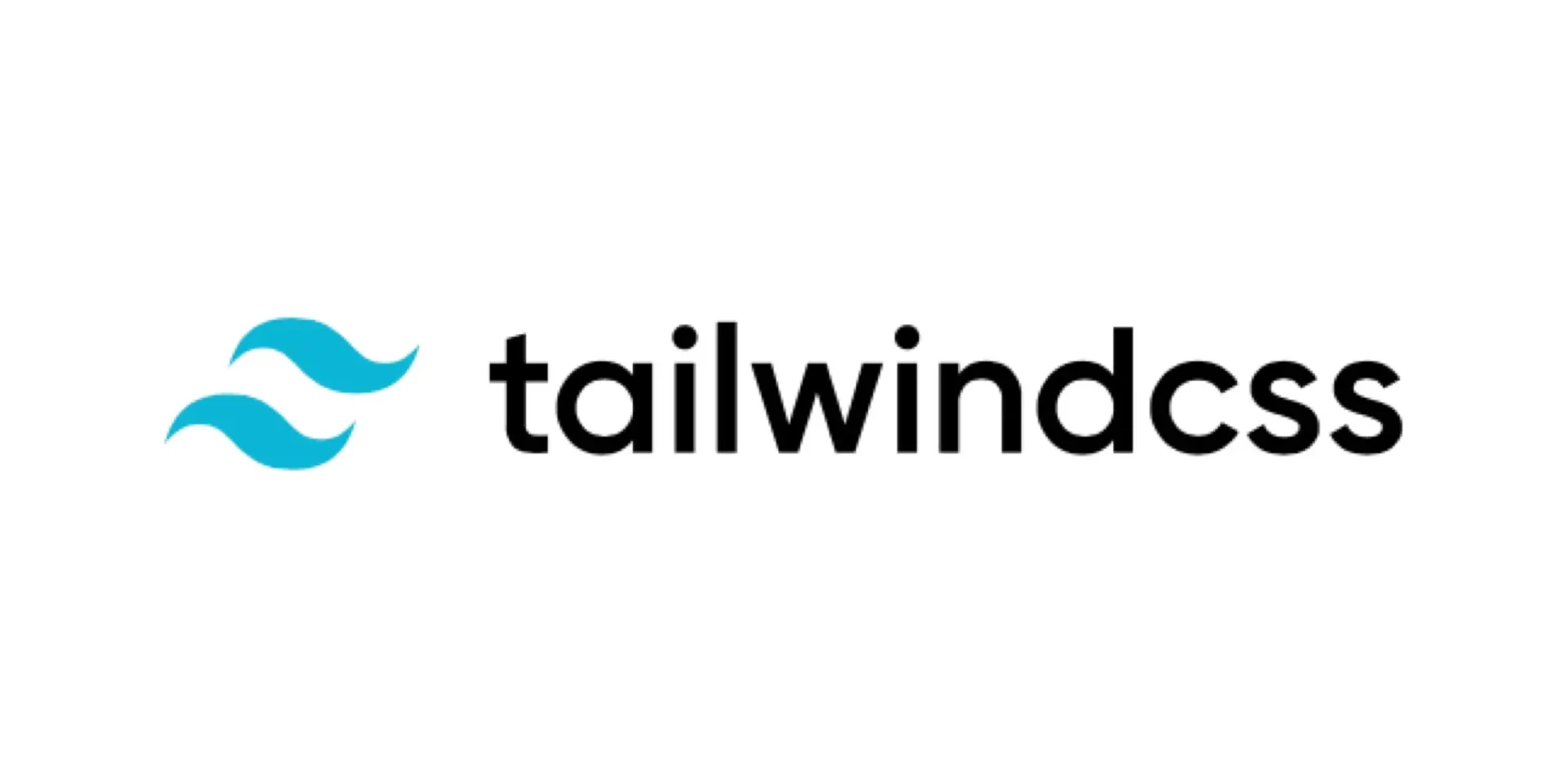
Install Tailwind CSS in your React project:
npm install tailwindcss postcss autoprefixer
npx tailwindcss init
Configure Tailwind to remove unused styles for production:
// tailwind.config.js
module.exports = {
purge: ['./src/**/*.{js,jsx,ts,tsx}', './public/index.html'],
theme: {},
variants: {},
plugins: [],
};
4. Establishing Global Styles
Create a global CSS file to include your base styles:
/* src/index.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
Include this file in your project’s entry point:
// src/index.js
import './index.css';
5. Implementation Best Practices
Performance Optimization
- Implement code-splitting for larger components
- Use React.memo for pure components
- Leverage useCallback and useMemo hooks appropriately
- Optimize Tailwind’s production build
Accessibility Considerations
- Implement ARIA labels and roles
- Ensure keyboard navigation
- Maintain sufficient color contrast
- Support screen readers
Testing Strategy
- Unit tests for individual components
- Integration tests for component combinations
- Visual regression testing
- Accessibility testing
Creating Reusable Components
With the foundation in place, let’s build reusable components.
1. Button Component
Buttons are essential elements in any design system. Here’s how to create a reusable button component:
Button.js
import React from 'react';
const Button = ({ children, variant = 'primary', onClick }) => {
const baseStyles = 'px-4 py-2 rounded font-semibold focus:outline-none';
const variants = {
primary: 'bg-primary text-white hover:bg-blue-700',
secondary: 'bg-secondary text-white hover:bg-gray-700',
accent: 'bg-accent text-black hover:bg-yellow-600',
};
return (
<button className={`${baseStyles} ${variants[variant]}`} onClick={onClick}>
{children}
</button>
);
};
export default Button;
Usage Example:
<Button variant="primary" onClick={() => alert('Clicked!')}>
Click Me
</Button>
2. Card Component
Cards are versatile and widely used UI elements.
Card.js
import React from 'react';
const Card = ({ title, children }) => {
return (
<div className="p-4 border rounded-lg shadow-md">
<h2 className="text-lg font-bold mb-2">{title}</h2>
<div>{children}</div>
</div>
);
};
export default Card;
Usage Example:
<Card title="Card Title">
<p>This is some card content.</p>
</Card>
Theming and Customization
A robust design system supports theming to adapt to different use cases. Tailwind’s configuration and React’s context API make theming seamless.
1. Dark Mode
Enable dark mode using Tailwind’s built-in support:
// tailwind.config.js
module.exports = {
darkMode: 'class',
theme: {},
};
Toggle dark mode in your components:
const App = () => {
return (
<div className="dark:bg-gray-800 dark:text-white">
<h1>Hello, World!</h1>
</div>
);
};
2. Using Context for Dynamic Themes
Set up a theme context to toggle between themes dynamically:
ThemeContext.js
import React, { createContext, useState } from 'react';
export const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
<div className={theme}>{children}</div>
</ThemeContext.Provider>
);
};
Usage in App.js
import React, { useContext } from 'react';
import { ThemeContext, ThemeProvider } from './ThemeContext';
const App = () => {
const { toggleTheme } = useContext(ThemeContext);
return (
<div>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
};
export default () => (
<ThemeProvider>
<App />
</ThemeProvider>
);
Documentation and Maintenance
Comprehensive Documentation Strategy
Component Documentation
Create detailed documentation for each component:
- Usage examples
- Props API
- Accessibility considerations
- Best practices
- Common pitfalls
Style Guide
Maintain a living style guide that includes:
- Design principles
- Component usage guidelines
- Visual language rules
- Brand voice and tone
Developer Guidelines
Establish clear guidelines for:
- Code style and conventions
- Component development process
- Testing requirements
- Performance standards
A design system is only as good as its documentation. Use tools like Storybook to create an interactive UI component library.
Setting Up Storybook
- Install Storybook:
npx storybook init
- Write stories for your components: Button.stories.js
import React from 'react';
import Button from './Button';
export default {
title: 'Components/Button',
component: Button,
};
export const Primary = () => <Button variant="primary">Primary Button</Button>;
- Run Storybook:
npm run storybook
Conclusion
Mastering design systems with React and Tailwind CSS is a journey that empowers you to deliver consistent, scalable, and visually appealing user experiences. By combining React’s component-based architecture with Tailwind’s utility-first approach, you can create systems that are both flexible and maintainable.
Remember that a successful design system is never truly finished – it should evolve with your product and team needs. Start small, build reusable components, and expand your system over time. With dedication and attention to detail, you’ll go from pixels to perfection in no time.
The key to success lies in finding the right balance between flexibility and consistency, between customization and standardization. By following the principles and practices outlined in this guide, you’ll be well-equipped to create and
READ MORE:
AI Integration Consulting for Your Business:Key Benefits
WebHook vs Pub/Sub: Key Differences and Best Use Cases