Table of Contents
React is a powerful JavaScript library for building user interfaces. To efficiently update the UI, React employs React Reconciliation and React Fiber, two core mechanisms that optimize rendering and improve performance. In this guide, we will explore what these concepts are, how they work, and their significance in React applications.
What is React Reconciliation?
Reconciliation is the process by which React updates the DOM to match the virtual DOM representation. When the state or props of a component change, React determines the minimal set of updates needed to efficiently update the UI instead of rendering everything from scratch.
How React Reconciliation Works
- Virtual DOM Comparison: React creates a virtual DOM, a lightweight representation of the actual DOM.
- Diffing Algorithm: When changes occur, React compares the new virtual DOM with the previous version using a diffing algorithm.
- Minimal Updates: React identifies the differences and updates only the affected parts of the actual DOM, improving performance.
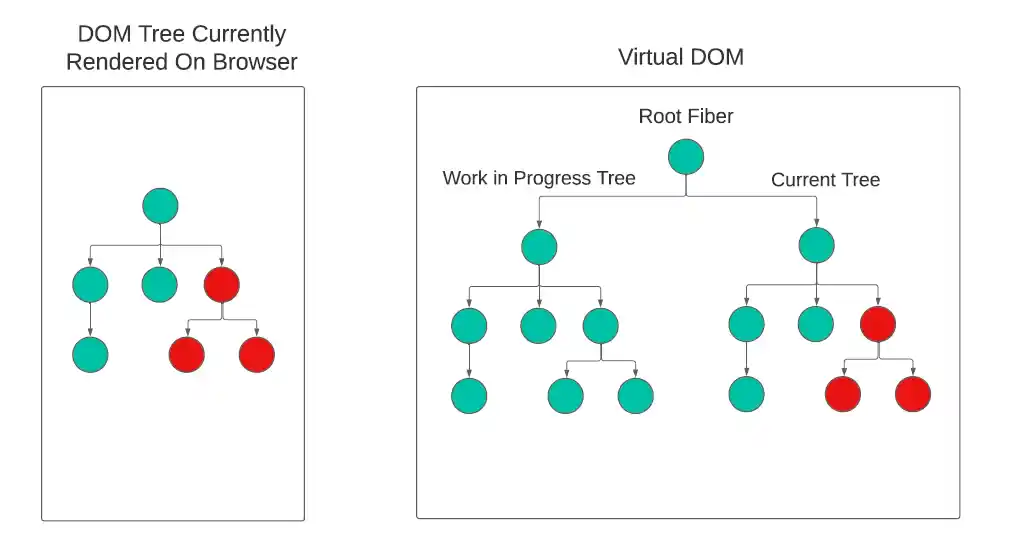
Optimizations in React Reconciliation (React Reconciliation has several optimizations that enhance performance)
- Keys in Lists: React uses keys to efficiently update lists and avoid unnecessary re-renders. Assigning unique keys to list items helps React identify which elements have changed, been added, or removed.
- Pure Components and
React.memo
: Helps prevent unnecessary re-renders by ensuring components update only when their props change. Pure components compare previous and new props, skipping updates if there are no changes. - shouldComponentUpdate Lifecycle Method: Allows developers to optimize updates by controlling when a component should re-render. By returning
false
when updates are unnecessary, developers can improve efficiency.
What is React Fiber?
React Fiber is a complete rewrite of the React reconciliation engine, introduced in React 16. It enhances React’s ability to handle complex UIs, animations, and concurrent rendering. React Reconciliation and React Fiber work together to ensure efficient rendering and smooth updates in modern applications.
Key Features of React Fiber
- Incremental Rendering: React Fiber breaks the rendering work into units and spreads them over multiple frames, improving responsiveness.
- Concurrency: Enables React to prioritize tasks, allowing smoother updates without blocking the UI.
- Pausing and Resuming Rendering: React Fiber can pause low-priority updates and resume them later, preventing UI freezes.
- Better Error Handling: Fiber improves error boundaries, allowing React to recover gracefully from rendering errors.
React Reconciliation and React Fiber
Feature | Legacy Reconciliation | React Fiber |
---|---|---|
Rendering Model | Synchronous | Asynchronous |
Performance | Limited Optimizations | High Performance |
Animation Handling | Poor Support | Smooth and Efficient |
Error Handling | Limited | Enhanced Recovery |
Benefits of React Reconciliation and React Fiber in Modern Applications
Modern applications demand high performance and smooth user experiences. React Reconciliation and React Fiber provide the following benefits:
- Improved Performance: By scheduling and prioritizing rendering work, React Fiber significantly improves app responsiveness. Users experience fewer lags and smoother UI transitions.
- Better User Experience: Smooth animations and transitions enhance user interactions. Whether users are scrolling, clicking, or navigating, the interface remains fluid.
- Optimized Large Applications: Fiber’s ability to pause and resume rendering makes it ideal for complex applications with heavy UI updates. Large applications often deal with multiple state updates simultaneously, and React Fiber helps manage them efficiently.
- Concurrent Mode and Suspense: Enables features like React Suspense and Concurrent Mode, improving data fetching and rendering efficiency. By allowing components to wait for asynchronous data before rendering, Suspense enhances the performance of server-side rendering (SSR) and client-side applications.
- Time-Slicing for Efficient Rendering: React Fiber utilizes time-slicing, which ensures that rendering is distributed over multiple frames. This approach prevents blocking the main thread and allows the UI to remain responsive even under heavy load.
- Streaming Server-Side Rendering (SSR): React Fiber enhances SSR by allowing React components to be streamed as they load. This means that users see content faster, even before the entire page has loaded.
When to Use React Fiber and React Reconciliation Strategies
React Reconciliation and React Fiber are already part of the React ecosystem, but knowing when to optimize specific features can improve performance:
- Use
React.memo
for Functional Components: If a component re-renders unnecessarily due to unchanged props, wrap it withReact.memo
to prevent redundant updates. - Leverage
useCallback
anduseMemo
: When passing functions as props, useuseCallback
to prevent unnecessary re-renders. Similarly, useuseMemo
to memoize expensive calculations. - Optimize Lists with Unique Keys: Always provide unique keys when rendering lists to help React efficiently track changes.
- Use Error Boundaries for Better Error Handling: Wrap critical UI components with error boundaries to catch and manage rendering errors.
- Enable Concurrent Mode for Better Performance: If your application handles complex UI interactions, enabling Concurrent Mode can significantly improve performance.
Conclusion
React Reconciliation and React Fiber are fundamental to React’s efficiency in updating the UI. Reconciliation ensures optimal DOM updates, while Fiber enhances performance through concurrent rendering. Understanding these concepts is crucial for building high-performance React applications with smooth user experiences.
By leveraging keys, React.memo
, and React Fiber’s concurrent rendering capabilities, developers can optimize their applications for better performance and scalability. These improvements make React a preferred choice for modern web development, ensuring that applications remain responsive and efficient.
For further reading, check out the official React documentation.