Table of Contents
Introduction to Flutter
Flutter is an open-source UI software development toolkit created by Google. It is designed to develop natively compiled applications for mobile (Android and iOS), web, and desktop platforms—all using a single codebase. Its hybrid nature allows developers to build efficient, scalable, and visually appealing applications while saving significant time and resources.
1. Single Codebase for All Platforms
With Flutter, developers write a single codebase that works seamlessly across Android, iOS, web, and desktop. This approach drastically reduces development and maintenance time while ensuring a consistent user experience across all platforms.
Example Code:
The following Flutter app will run on Android, iOS, web, and desktop without any modification:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Flutter App')),
body: Center(child: Text('Hello, World!')),
),
);
}
}
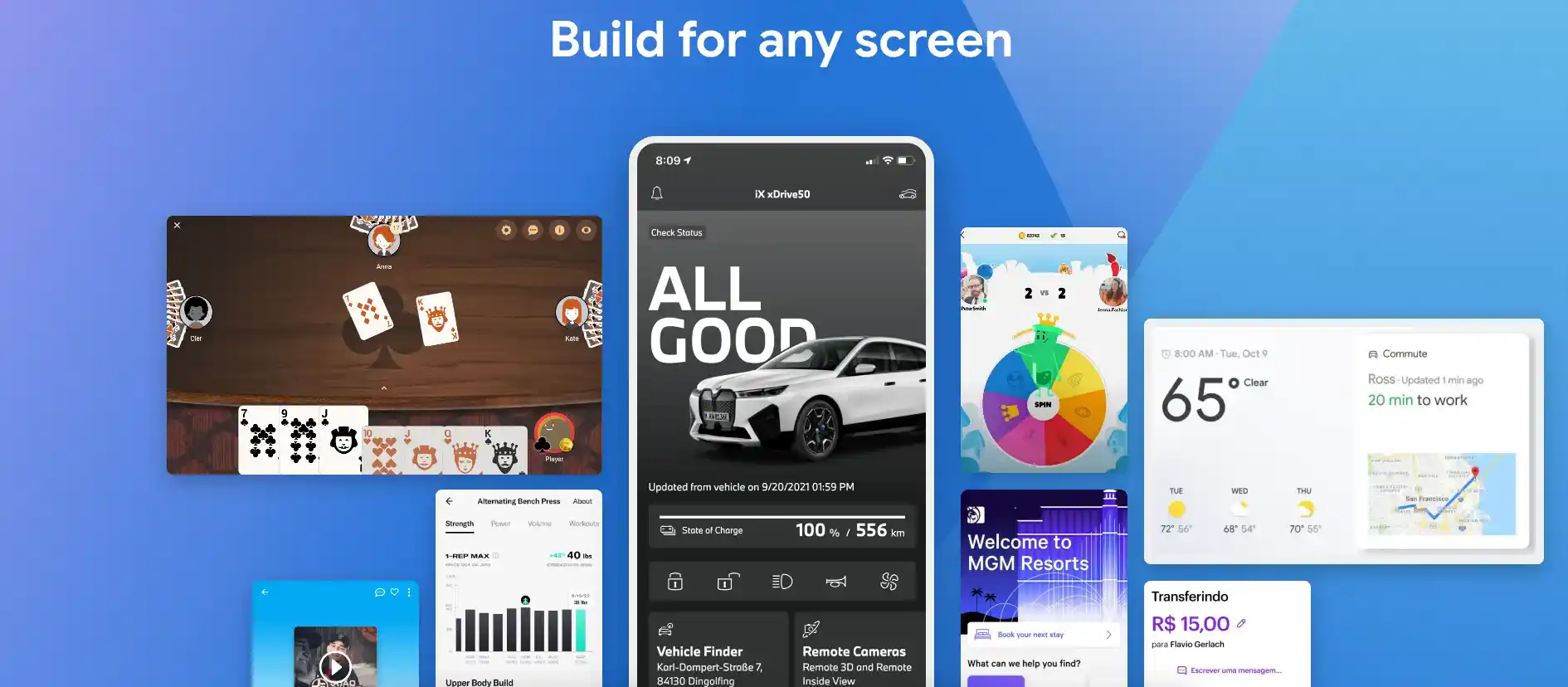
2. Hot Reload for Faster Development
Flutter’s hot reload allows developers to see the results of code changes instantly, without restarting the app. This feature significantly improves productivity and makes experimenting with UI and functionality much easier.
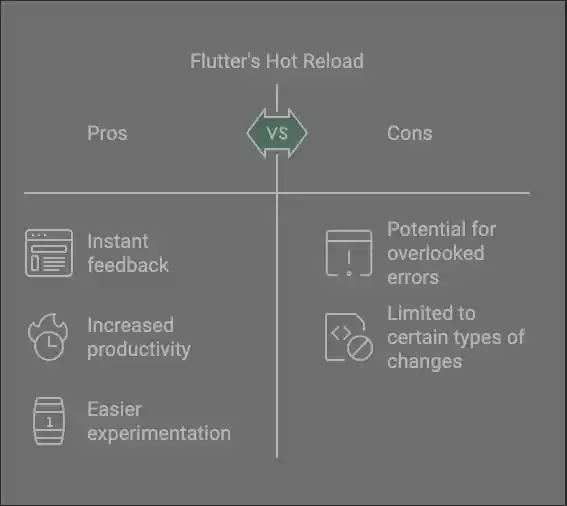
3. Extensive Pre-Built Widgets
It offers an extensive library of pre-built widgets that conform to both Material Design (for Android) and Cupertino Design (for iOS). These widgets are highly customizable, ensuring a consistent and polished UI for any app.
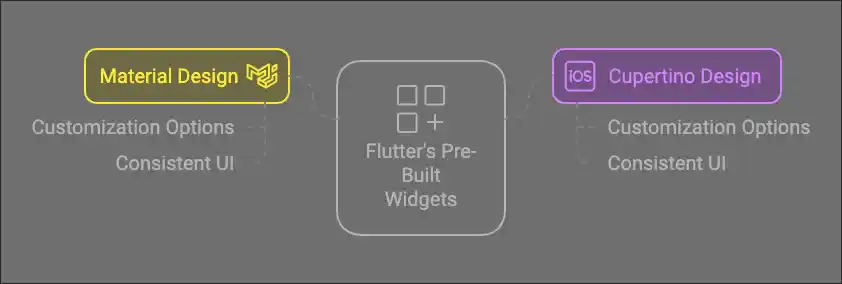
Example of Using a Pre-Built Button Widget:
ElevatedButton(
onPressed: () {
print('Button Pressed!');
},
child: Text('Click Me'),
)
4. Native Performance
Unlike traditional hybrid frameworks that use a JavaScript bridge, Flutter apps compile directly to native machine code using Dart. This approach delivers smooth animations, faster load times, and high-performance UI interactions.
5. Open-Source Ecosystem with Strong Community Support
It is open-source and backed by a vibrant community of developers. With numerous packages, plugins, and forums, developers can easily find solutions to challenges and access resources to enhance their apps.
6. Customizable Animations and Graphics
Flutter leverages the Skia rendering engine to create custom animations and visually rich UIs. Whether you’re building a game or a business app, Flutter’s robust animation toolkit allows you to craft dynamic and engaging user experiences.
Example of a Simple Animation:
import 'package:flutter/material.dart';
class AnimatedBox extends StatefulWidget {
@override
_AnimatedBoxState createState() => _AnimatedBoxState();
}
class _AnimatedBoxState extends State<AnimatedBox> {
double _size = 100;
void _toggleSize() {
setState(() {
_size = _size == 100 ? 200 : 100;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Animated Box')),
body: Center(
child: GestureDetector(
onTap: _toggleSize,
child: AnimatedContainer(
duration: Duration(seconds: 1),
width: _size,
height: _size,
color: Colors.blue,
),
),
),
);
}
}
void main() => runApp(MaterialApp(home: AnimatedBox()));
7. Responsive Design for Multiple Devices
Flutter makes it easy to design apps that look great and function seamlessly across any device, whether it’s a smartphone, tablet, or desktop.
- Responsive Layout Builder: With tools like
LayoutBuilder
andMediaQuery
, Flutter allows developers to create dynamic layouts that adapt to different screen sizes and orientations. These tools enable the app to adjust its UI elements based on the available screen space. - Adaptive Widgets: Flutter provides adaptive widgets like
AdaptiveLayout
and platform-specific UI packages to ensure the app feels natural on both iOS and Android. Widgets automatically adjust their styles and behavior to align with the platform’s design guidelines. - Flexibility with Grid and Flex Layouts: Flutter’s
Flex
andGridView
widgets offer powerful mechanisms for designing complex, responsive UIs. Developers can easily create layouts that rearrange or resize elements based on the screen dimensions. - Device-Specific Customization: With conditional logic, developers can implement device-specific layouts or features, such as splitting views for larger screens like tablets or desktops while maintaining a unified codebase.
This robust support for responsive design ensures that Flutter apps deliver a consistent user experience across various devices, enhancing user satisfaction and engagement.
Example of Responsive Layout:
class ResponsiveWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth > 600) {
return Text('Tablet or Desktop Layout');
} else {
return Text('Mobile Layout');
}
},
);
}
}
8. Cross-Platform Backend Integration
Flutter excels in providing seamless integration with a wide range of backend services, making it an ideal choice for building scalable, full-stack applications.
- Firebase Integration: Flutter’s extensive support for Firebase services enables developers to integrate real-time databases, authentication, cloud storage, push notifications, and analytics with minimal effort. Firebase plugins, such as
cloud_firestore
andfirebase_auth
, streamline backend connectivity and reduce development time. - REST API Support: Flutter simplifies communication with RESTful APIs using libraries like
http
anddio
. These libraries provide robust tools for making network requests, handling JSON responses, and ensuring secure communication with backend servers. - GraphQL Support: Flutter offers efficient integration with GraphQL APIs using packages like
graphql_flutter
andhasura_connect
. These tools enable developers to fetch, cache, and update data seamlessly, providing efficient and optimized data handling. - Custom Backend Solutions: Whether it’s Node.js, Django, or Spring Boot, Flutter can connect to any custom backend solution. By leveraging native HTTP requests or third-party libraries, developers can easily manage API endpoints and data exchange.
This rich backend integration capability makes Flutter a comprehensive solution for creating cross-platform applications that cater to diverse business needs, whether it’s a small-scale app or an enterprise-grade system.
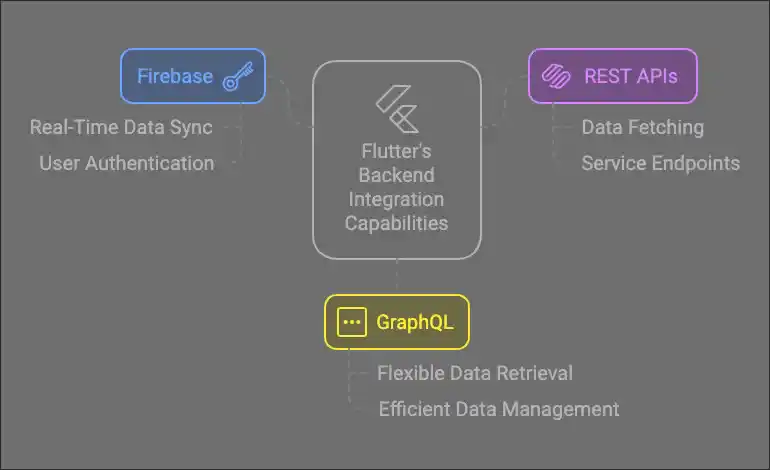
Example of Fetching Data from an API:
import 'package:flutter/material.dart';
import 'dart:convert';
import 'package:http/http.dart' as http;
class FetchDataExample extends StatelessWidget {
Future<List<dynamic>> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
return json.decode(response.body);
} else {
throw Exception('Failed to load data');
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Fetch Data Example')),
body: FutureBuilder<List<dynamic>>(
future: fetchData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return Center(child: CircularProgressIndicator());
} else if (snapshot.hasError) {
return Center(child: Text('Error: ${snapshot.error}'));
} else {
return ListView.builder(
itemCount: snapshot.data!.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(snapshot.data![index]['title']),
subtitle: Text(snapshot.data![index]['body']),
);
},
);
}
},
),
);
}
}
void main() => runApp(MaterialApp(home: FetchDataExample()));
9. Efficient State Management
State management is a critical aspect of app development, and Flutter provides a variety of tools and libraries to handle state effectively. These tools simplify managing and synchronizing the state of the UI and application logic, making it easier to build scalable and maintainable apps.
For complex applications requiring advanced control, Bloc or Redux can handle intricate workflows efficiently.
- Built-In State Management: Flutter comes with built-in solutions for state management, such as
setState
for managing local widget state andInheritedWidget
for propagating state down the widget tree. While suitable for smaller apps, these methods can become cumbersome in complex applications. - Popular State Management Libraries: Flutter’s ecosystem offers powerful libraries for more advanced state management:
- Provider: A lightweight and flexible library endorsed by Google. It simplifies state management by using dependency injection and context to pass state across the widget tree.
- Performance Optimization: Efficient state management tools ensure that only necessary widgets rebuild, minimizing performance overhead. For example, by using fine-grained state listeners in libraries like Riverpod or Bloc, developers can avoid unnecessary UI re-renders, leading to smoother user experiences.
- Scalability: With solutions like Provider or Bloc, it’s easier to manage state in large-scale applications. These tools provide patterns and best practices for managing both UI and application-wide state, ensuring maintainability as the app grows.
- Flexibility for Different Use Cases: Flutter’s state management options cater to various use cases:
- For simple state,
setState
orInheritedWidget
might suffice. - For medium-complexity apps, Provider or Riverpod offers simplicity with scalability.
- For simple state,
10. Future-Proof and Backed by Google
As a Google product, Flutter benefits from continuous updates and long-term support. It’s also increasingly being adopted by enterprises, ensuring its relevance and growth in the development ecosystem.
Disadvantages of Flutter
While It offers many advantages, it’s important to consider its limitations:
- Large App Size: Flutter apps are typically larger than their native counterparts. This is because the This framework includes the Dart runtime, rendering engine, and other libraries in the final app package, increasing its size.
- Limited Platform-Specific Features: Accessing advanced native features may require developing custom plugins or using platform-specific code. This can increase development complexity and effort, especially for features not yet supported by Flutter’s core libraries.
- Complex Debugging: Debugging platform-specific issues can be challenging. While It offers tools like DevTools, solving bugs that arise from communication between the this framework and native code may require deeper expertise in both Flutter and native development.
- Limited Ecosystem for Third-Party Libraries: Although the Flutter ecosystem is growing, it still lags behind native ecosystems like Android and iOS in terms of the availability and maturity of third-party libraries. Developers may need to create custom solutions for certain features.
- Performance Constraints for Complex UI Animations: While It excels at creating beautiful UIs, extremely complex animations or graphic-intensive apps may experience performance bottlenecks compared to highly optimized native implementations.
- Dependence on Google: It is developed and maintained by Google. While this ensures high-quality updates, it also poses a risk, as any strategic shift by Google could impact it’s future.
Conclusion
Flutter is a transformative framework for modern app development, enabling developers to create high-performance, cross-platform applications efficiently. Its hybrid nature, coupled with robust tools and community support, makes it a strong contender for businesses and developers looking to streamline their workflows.
Despite some drawbacks, its benefits make it a top choice for versatile software development in today’s competitive landscape.