Table of Contents
In today’s world of massive data, ensuring fast and efficient data handling is crucial. Bloom filters are an elegant solution to this challenge. They are a simple, space-efficient probabilistic data structure used to test whether an element is a member of a set. While they may allow false positives, they never produce false negatives, making them a powerful pre-check mechanism for filtering data before querying a database.
This article will explain Bloom filters in depth, covering their workings, advantages, limitations, and practical implementation in Python. We will also explore concepts like true positives and false negatives, ensuring a solid grasp of this efficient filtering technique.
What is a Bloom Filter?
A Bloom filter is a binary array of size m
, initialized with all bits set to 0. It uses k
independent hash functions to map elements to positions in the array. The primary operations supported are:
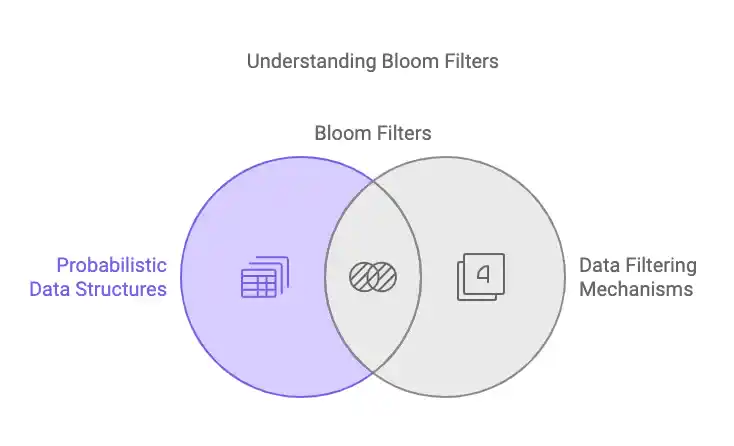
- Insert: Add an element to the filter by hashing it
k
times and setting the corresponding bit positions in the array to 1. - Check: Verify if an element might exist by checking the bit positions indicated by the
k
hash functions. If all positions are 1, the element might exist; if any position is 0, the element definitely does not exist.
Key Features of Bloom Filters
- Space Efficiency: They use less memory compared to other data structures like hash tables.
- Speed: Operations are fast with
O(k)
complexity for both insert and lookup. - False Positives: A query may falsely indicate that an element exists, but this probability can be minimized by tuning
m
andk
. - No False Negatives: If the filter says an element doesn’t exist, it’s guaranteed to be true.
Mathematical Insights
The probability of a false positive is given by:
Where:
n
= Number of elements added to the filterm
= Size of the bit arrayk
= Number of hash functions
The optimal number of hash functions to minimize the false positive rate is:
This mathematical foundation ensures that Bloom filters are highly tunable for specific use cases.
True Positives and False Negatives
- True Positive: The filter correctly identifies that an element exists.
- False Positive: The filter indicates an element exists when it does not. This is a trade-off for space efficiency.
- False Negative: Bloom filters avoid this scenario entirely. If an element is not in the filter, it is guaranteed not to be in the set.
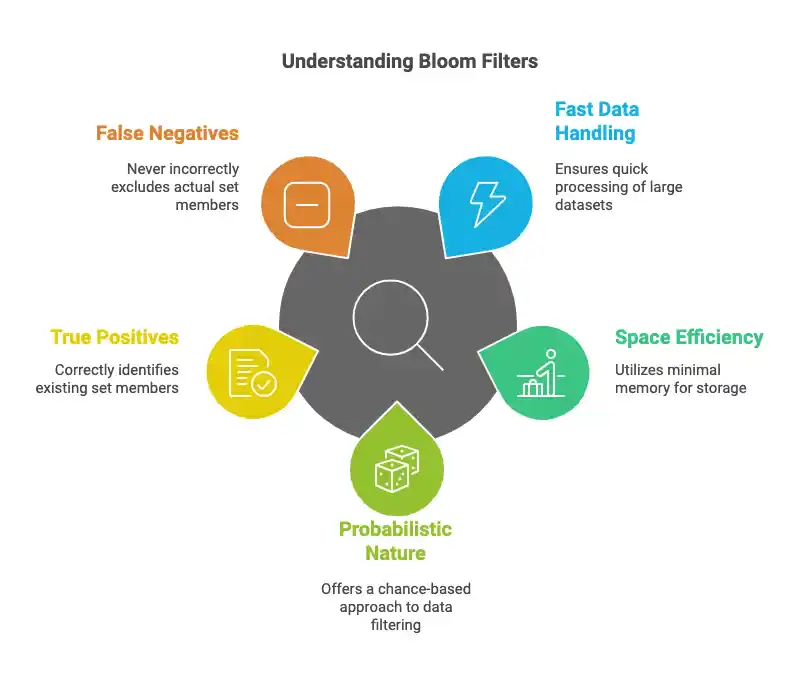
Applications of Bloom Filters
- Databases: To avoid unnecessary disk reads by pre-checking if a key exists.
- Web Caching: To test whether a URL is already cached.
- Distributed Systems: To synchronize nodes by determining overlapping data.
- Blockchain: For lightweight clients to query specific transactions.
- Email Spam Filters: To quickly check if an email is from a known spam source.
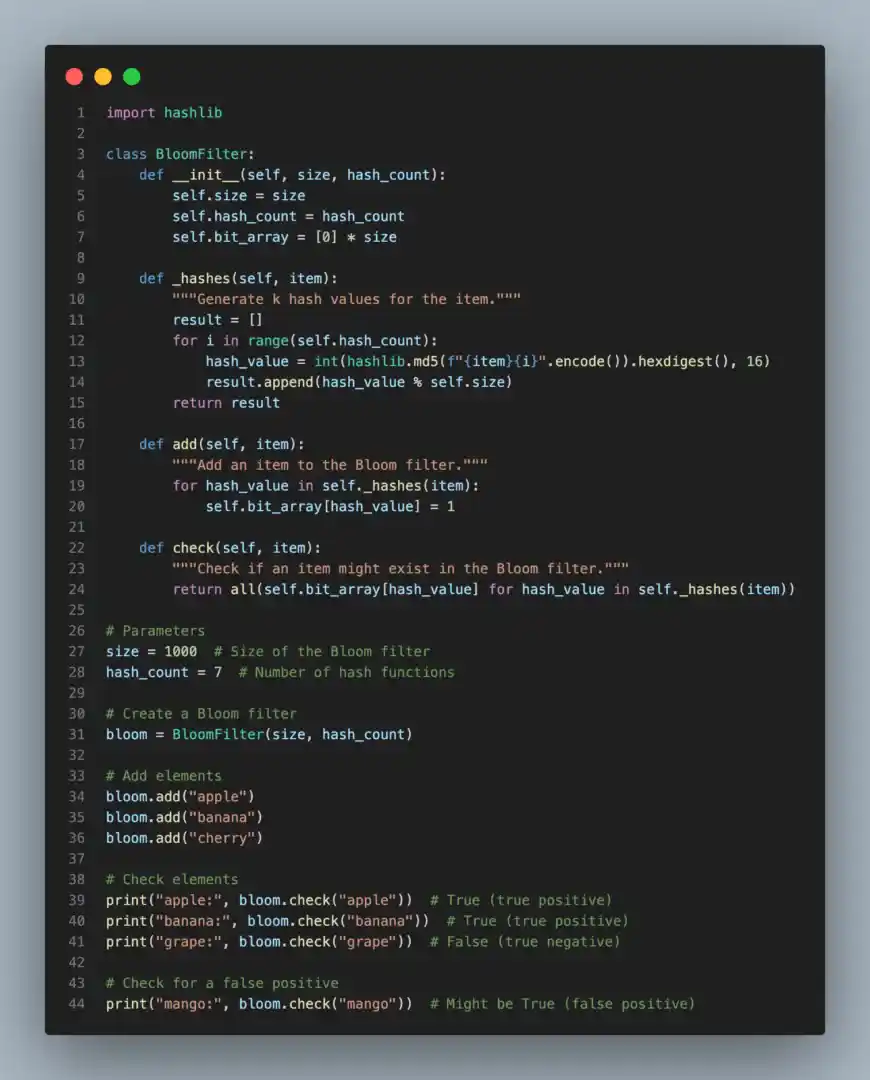
Python Implementation of a Bloom Filter
Here’s a Python implementation of a simple Bloom filter using the hashlib
library for hashing:
Tuning the Bloom Filter
To optimize the false positive rate:
Choose m
(size of the bit array) proportional to n
(number of items).
Use an optimal k
(number of hash functions) calculated as:
For instance, if n = 1000
and you want a false positive rate of 1%
, you can calculate m
and k
accordingly.
Advantages and Limitations
Advantages:
- Space-efficient: Requires significantly less memory
- Fast: Performs membership checks in constant time.
- No false negatives: Guarantees accuracy when reporting non-existence.
Limitations:
- False positives: Introduces uncertainty in membership.
- Fixed size: Requires resizing and rebuilding for growing datasets.
- No deletions: Cannot remove items (though Counting Bloom Filters address this).
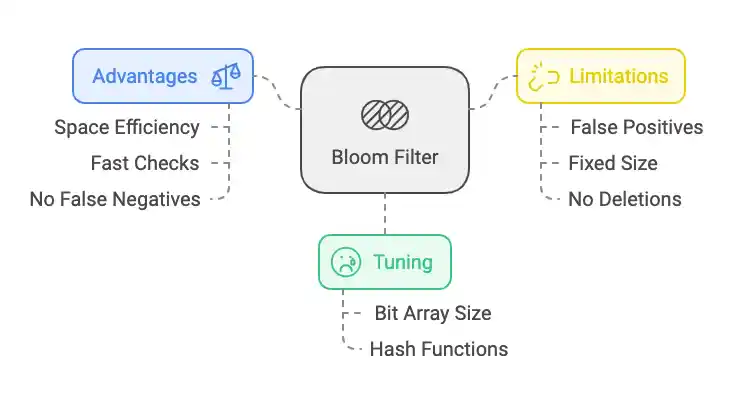
Conclusion
Bloom filters are a simple yet powerful technique for pre-checking the existence of data in large datasets. Their efficiency and minimal memory usage make them an essential tool in modern computing, particularly in databases and distributed systems. By understanding their inner workings and applying them correctly, you can significantly optimize your applications.
Would you like to explore advanced topics like Counting Bloom Filters, Scalable Bloom Filters, or their application in distributed systems? Let us know!
READ MORE:
Odoo: 5 Strategies to Boost Your Business Growth
Ground Breaking Power of AI-Driven Climate Models in Disaster Prevention