Table of Contents
In this fast-moving landscape of web development, choosing the appropriate front-end framework makes all the difference in the success of your project. This blog contrasts and compares Angular vs React, two popular front-end frameworks, for different features so that developers can make an informed decision about which one would suit them best.
Angular Overview
Angular is a comprehensive, open-source front-end framework developed by Google, primarily used for building dynamic, single-page web applications (SPA) and enterprise-level applications.
Introduced in 2010, Angular is built on TypeScript and follows the Model-View-Controller (MVC) architecture, ensuring a clean separation of concerns.
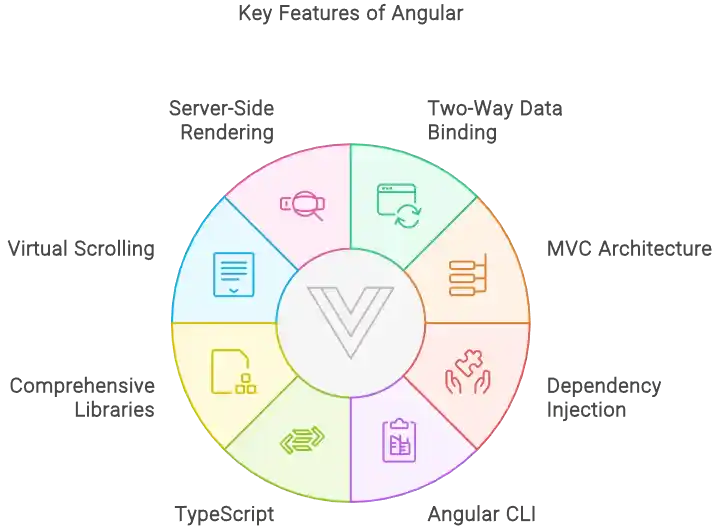
Key Features of Angular:
- Two-Way Data Binding: Synchronizes data between the model and the view in real-time, making the development process more straightforward.
- MVC Architecture: Promotes organized and maintainable code by separating the application logic, user interface, and data.
- Dependency Injection: Enhances modularity and facilitates the management of dependencies within the application.
- Angular CLI: A powerful Command Line Interface that simplifies the management, scaffolding, and maintenance of Angular projects.
- TypeScript: Utilizes TypeScript, which allows for the writing of more robust and maintainable code.
- Comprehensive Libraries: Includes built-in libraries for routing, form management, HTTP client, and more, speeding up the development process.
- Virtual Scrolling: Efficiently handles large lists of data by rendering only the visible items, improving performance.
- Server-Side Rendering: Supports server-side rendering, enhancing the performance and SEO of the application.
These features make Angular a powerful and versatile framework, suitable for developing large-scale applications that require high performance and maintainability.
React Overview
React is an open-source JavaScript library developed by Facebook for building user interfaces, particularly for single-page applications where data changes frequently.
Introduced in 2013, React allows developers to create reusable UI components, making it highly efficient for developing dynamic and interactive web applications.
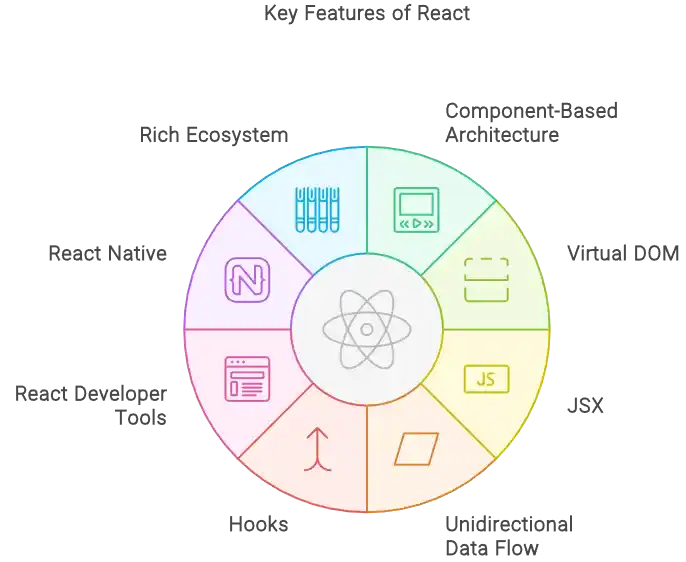
Key Features of React:
- Component-Based Architecture: Encourages the creation of reusable and independent components, which simplifies development and enhances maintainability.
- Virtual DOM: Uses a virtual DOM to optimize rendering by updating only the parts of the UI that have changed, resulting in improved performance.
- JSX: Combines JavaScript and HTML-like syntax, making it easier to write and understand the structure of UI components.
- Unidirectional Data Flow: Ensures a predictable data flow, which simplifies debugging and state management.
- Hooks: Allows the use of state and other React features in functional components, making it easier to manage state and lifecycle events.
- React Developer Tools: Provides powerful tools for debugging and inspecting React applications within the browser.
- React Native: Enables the development of cross-platform mobile applications using the same React principles, extending its reach beyond the web.
- Rich Ecosystem: Supported by a vast ecosystem of libraries and tools, such as Redux for state management and React Router for navigation.
These features make React a popular choice for developers looking to build high-performance, scalable, and maintainable web applications.
Key Differences Between Angular and React
1. Learning Curve
Understanding the complexity and learning curves of Angular and React can help developers choose the right framework based on their experience and project requirements.
Angular
Complexity:
- Comprehensive Framework: Angular is a fully-fledged framework that offers a wide range of built-in features, which can be overwhelming for beginners.
- TypeScript: Angular is built with TypeScript, adding another layer of complexity for those unfamiliar with it.
- Strict Structure: Follows a strict project structure and design patterns, which can be both a strength and a hurdle depending on the developer’s familiarity with these patterns.
Learning Curve:
- Steep Initial Learning Curve: Due to its comprehensive nature and the need to learn TypeScript, Angular has a steeper initial learning curve.
- Extensive Documentation: Angular offers extensive documentation and a large community, which can aid in overcoming the initial complexity.
- Long-Term Benefits: Once mastered, Angular’s robust features and structure can lead to more maintainable and scalable applications.
React
Complexity:
- Library, Not a Framework: React is a library focused on building UI components, which means it is less opinionated and more flexible but requires additional libraries for state management, routing, etc.
- JavaScript and JSX: React uses JSX, a syntax extension that combines JavaScript and HTML, which can be simpler for developers familiar with JavaScript.
Learning Curve:
- Gentler Initial Learning Curve: React’s simplicity and focus on UI components make it easier to pick up initially, especially for those with JavaScript experience.
- Incremental Learning: Developers can start with basic components and gradually learn more advanced concepts like state management and hooks.
- Rich Ecosystem: The extensive ecosystem and community support make it easier to find resources and tools, aiding the learning process.
Angular may present a steeper learning curve initially due to its comprehensive nature and use of TypeScript but offers a structured approach that can lead to highly maintainable applications.
On the other hand, React provides a gentler entry point with its simpler, component-based approach and strong ecosystem, making it a popular choice for developers who prefer flexibility and gradual learning.
2. Syntax and Approach
Understanding the syntax and overall approach of Angular and React can help in choosing the right framework based on your development style and project needs.
Angular
Syntax:
- TypeScript: Angular uses TypeScript, which is a statically typed superset of JavaScript. This adds type safety and can make the code more robust and maintainable.
- Decorators: Angular uses decorators to define components, services, and other elements. For example, @Component is used to define a component.
- Templates: Angular templates use a specific syntax for data binding, such as {{ }} for interpolation and [(ngModel)] for two-way binding.
Overall Approach:
- Opinionated Framework: Angular provides a comprehensive set of tools and follows a strict project structure, which can streamline development but may feel rigid to some developers.
- MVVM Architecture: Follows the Model-View-ViewModel (MVVM) design pattern, encouraging a clear separation of concerns.
- Dependency Injection: A built-in dependency injection system promotes modular and testable code.
- Comprehensive Tooling: Angular CLI (Command Line Interface) offers extensive tooling for project setup, testing, and deployment.
Example of an Angular Component:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `<h1>{{ title }}</h1>`,
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Hello Angular';
}
React
Syntax:
- JavaScript and JSX: React uses JavaScript along with JSX, a syntax extension that allows HTML-like code within JavaScript, making it intuitive for those familiar with JavaScript.
- Functional and Class Components: React allows the creation of components using both functional and class-based approaches, though functional components with hooks are now more common.
- JSX Expressions: Uses curly braces {} to embed JavaScript expressions within JSX.
Overall Approach:
- Library, Not a Framework: React is focused on building UI components and is less opinionated, offering more flexibility but requiring additional libraries for state management, routing, etc.
- Component-Based Architecture: Emphasizes reusable, independent components, making the development process modular and maintainable.
- Unidirectional Data Flow: Promotes predictable state management with a one-way data flow.
- Hooks: Introduced to manage state and lifecycle events within functional components, making them more powerful and easier to work with.
Example of a React Component:
import React, { useState } from 'react';
function App() {
const [,title setTitle] = useState('Hello React');
return (
<div>
<h1>{title}</h1>
</div>
);
}
export default App;
Angular offers a more structured and opinionated approach with TypeScript and MVVM architecture, which can be beneficial for large-scale applications.
React, on the other hand, provides greater flexibility with its component-based architecture and the use of JSX, making it a popular choice for developers who prioritize simplicity and modularity.
3. Performance Characteristics
Performance is a crucial factor when choosing a front-end framework, as it directly affects the user experience and responsiveness of applications. Let’s compare the performance characteristics of Angular and React.
Angular
Performance Characteristics:
- Two-Way Data Binding: Angular’s two-way data binding can sometimes lead to performance bottlenecks, especially in large applications, as it requires more processing to keep the model and view in sync.
- Digest Cycle: Angular uses a digest cycle to check for changes in the application state, which can become inefficient with a large number of watchers and bindings.
- Ahead-of-Time (AOT) Compilation: Angular provides an AOT compilation feature, which compiles the application during the build process. This reduces the amount of JavaScript that needs to be parsed and executed in the browser, leading to faster load times and improved performance.
- Tree Shaking: Angular’s build process includes tree shaking, which eliminates unused code, reducing the final bundle size and improving load times.
- Change Detection: Angular has an efficient change detection mechanism, but it can still be less performant compared to React in certain scenarios with large and complex applications.
Performance Optimization:
- OnPush Change Detection: Developers can use the OnPush change detection strategy to manually control when components need to be checked for changes, improving performance.
- Lazy Loading: Angular supports lazy loading of modules, which allows parts of the application to be loaded only when needed, reducing initial load time.
React
Performance Characteristics:
- Virtual DOM: React uses a virtual DOM to manage updates efficiently. The virtual DOM minimizes direct manipulation of the real DOM, resulting in better performance, especially for dynamic and frequently updated interfaces.
- Reconciliation Algorithm: React’s reconciliation algorithm (also known as the diffing algorithm) efficiently updates only the parts of the DOM that have changed, reducing the overall rendering time.
- Component-Based Rendering: React’s component-based architecture allows for fine-grained control over rendering, enabling developers to optimize performance at the component level.
Performance Optimization:
- Memoization and useMemo: React offers memorization techniques, such as React.memo and the useMemo hook, to prevent unnecessary re-renders of components.
- Code Splitting and Lazy Loading: React supports code splitting and lazy loading with tools like Webpack, which can help reduce the initial load time by loading only the necessary code chunks.
- Concurrent Mode: React’s upcoming Concurrent Mode aims to improve the performance of complex applications by making rendering more responsive and interruptible.
Angular provides robust performance features such as AOT compilation and tree shaking, but its two-way data binding and digest cycle can introduce performance challenges in large applications.
React, with its virtual DOM and efficient reconciliation algorithm, generally offers better performance for dynamic and frequently updated interfaces.
Both frameworks provide various optimization techniques to help developers achieve the best possible performance for their applications.
4. Tooling and Ecosystem Support
Both Angular and React have strong tooling and ecosystem support, but they differ in their approaches and the breadth of their ecosystems.
Let’s evaluate the tooling and ecosystem support for each.
Angular
Tooling:
- Angular CLI: It provides a powerful Command Line Interface (CLI) that simplifies project setup, development, testing, and deployment. It offers commands to generate components, services, modules, and more, streamlining the development process.
- Integrated Development Environment (IDE) Support: Angular has strong support in popular IDEs like Visual Studio Code, WebStorm, and IntelliJ IDEA, with features like IntelliSense, debugging, and TypeScript support.
- Built-In Tools: It includes built-in tools for unit testing (Karma) and end-to-end testing (Protractor), making it easier to maintain code quality and reliability.
Ecosystem Support:
- Comprehensive Framework: Angular is a comprehensive framework that includes many built-in features like routing, form validation, HTTP client, and more, reducing the need for third-party libraries.
- Official Libraries: Angular provides official libraries and modules for various functionalities, ensuring compatibility and long-term support.
- Community and Resources: Angular has a vibrant community and extensive documentation, tutorials, and resources available, helping developers quickly find solutions and best practices.
React
Tooling:
- Create React App: React offers Create React App, a CLI tool that sets up a new React project with a standardized configuration, including support for modern JavaScript features, CSS, and more.
- IDE Support: React is well-supported in popular IDEs like Visual Studio Code, WebStorm, and Atom, with extensions and plugins for JSX, React-specific linting, and debugging.
- Third-Party Tools: React relies on third-party tools for tasks like state management (Redux, MobX), routing (React Router), and testing (Jest, Enzyme), providing flexibility but requiring additional setup.
Ecosystem Support:
- Rich Ecosystem: React has a vast ecosystem with a plethora of third-party libraries and tools, allowing developers to choose the best solutions for their specific needs.
- Modularity: React’s modularity means that developers can pick and choose libraries for different functionalities, leading to a highly customizable development stack.
- React Native: React extends beyond web development with React Native, enabling developers to build cross-platform mobile applications using the same principles and components.
- Community and Resources: React has a large and active community, with abundant resources, tutorials, and third-party libraries available, making it easy to help find and solutions.
Angular offers a more integrated and opinionated toolset with its robust CLI and built-in tools, making it easier to get started with a standardized setup.
React provides greater flexibility and a rich ecosystem of third-party tools and libraries, allowing for a highly customizable development experience.
Both frameworks have strong community support and extensive resources, ensuring that developers can find the help they need.
5. Scalability of Angular and React for Large, Enterprise-Level Applications
When it comes to building large, enterprise-level applications, both Angular and React offer features that support scalability, but they do so in different ways. Let’s discuss the scalability aspects of each framework.
Angular
Scalability Features:
- Modular Architecture: Angular’s modular architecture encourages splitting the application into modules, making it easier to manage, maintain, and scale. Modules can be lazy-loaded to improve performance by loading only the necessary parts of the application when needed.
- TypeScript: It is built with TypeScript, which provides static typing, interfaces, and decorators. These features help catch errors early, enforce coding standards, and improve code maintainability, which is crucial for large applications.
- Dependency Injection: Angular’s built-in dependency injection system promotes the development of modular, testable, and scalable code by managing dependencies efficiently.
- Comprehensive Tooling: Angular CLI automates many repetitive tasks, such as project setup, testing, and deployment, which can significantly improve productivity in large projects.
- Enterprise-Level Support: Angular is backed by Google and has long-term support (LTS) plans, ensuring stability and regular updates, which is essential for enterprise applications that require long-term maintenance.
Scalability in Practice:
- Structured Approach: Angular’s opinionated and structured approach can be beneficial for large teams, as it enforces a consistent coding style and project structure.
- Built-In Features: With built-in features like routing, form validation, and HTTP client, Angular reduces the need for third-party libraries, simplifying dependency management and enhancing scalability.
React
Scalability Features:
- Component-Based Architecture: React’s component-based architecture allows for the creation of reusable, self-contained components. This promotes code reuse and easier maintenance, which are critical for scaling applications.
- Flexibility: React’s flexibility allows developers to choose the best libraries and tools for their specific needs, enabling them to build a scalable architecture tailored to their requirements.
- State Management: React’s ecosystem includes robust state management libraries like Redux and MobX, which help manage application state in a scalable manner.
- Code Splitting: React supports code splitting and lazy loading through tools like Webpack and React’s built-in React.lazy, improving performance by loading only the necessary code chunks.
- Server-Side Rendering (SSR): React’s support for server-side rendering (with frameworks like Next.js) can improve performance and SEO, which is beneficial for large-scale applications.
Scalability in Practice:
- Customizability: React’s unopinionated nature allows for a highly customizable architecture, letting developers choose the best patterns and practices for scaling their applications.
- Ecosystem: The vast ecosystem of third-party libraries and tools provides developers with numerous options to extend and scale their applications.
- Community and Resources: React’s large community and extensive resources mean that developers can find solutions and best practices for scaling their applications.
Angular offers a more structured and opinionated approach with built-in features and strong tooling, making it well-suited for large, enterprise-level applications that benefit from a consistent coding style and project structure.
React, on the other hand, provides greater flexibility and a component-based architecture that promotes code reuse and scalability.
Both frameworks have robust features and ecosystem support that make them capable of handling large-scale, enterprise-level applications effectively.
6. Security Features
Security is a crucial aspect of web development, and both Angular and React offer features to help developers build secure applications. Here’s a comparison of the security features provided by each framework.
Angular
Security Features:
- Built-In Sanitization: It automatically sanitizes untrusted values in templates to prevent Cross-Site Scripting (XSS) attacks. This is done by default for data binding expressions and template values.
- Content Security Policy (CSP): Angular supports CSP, which helps to mitigate XSS and other attacks by specifying which content is allowed to be loaded and executed in the application.
- HTTP Client Security: Angular’s HttpClient module includes built-in features to guard against attacks, such as CSRF (Cross-Site Request Forgery) protection by using XSRF tokens.
- Strict Contextual Escaping (SCE): Angular enforces SCE to prevent the execution of potentially dangerous code by restricting the contexts in which bindings can occur.
- Dependency Injection: Angular’s dependency injection system can help in managing and securing dependencies effectively, reducing the risk of injecting malicious code.
Best Practices:
- Use Angular’s built-in sanitization and SCE to prevent XSS attacks.
- Implement CSP headers to restrict the sources of content that can be loaded.
- Use Angular’s HttpClient with XSRF protection for secure API communication.
- Regularly update Angular and its dependencies to include the latest security patches.
React
Security Features:
- JSX Escaping: React automatically escapes any values embedded in JSX before rendering them, preventing XSS attacks by default.
- Dangerously Set InnerHTML: React provides the dangerouslySetInnerHTML attribute for injecting HTML into the DOM, but it should be used cautiously and only with trusted content to avoid XSS vulnerabilities.
- Content Security Policy (CSP): React supports CSP to help prevent XSS and other attacks by defining which content sources are permissible.
- State Management Security: Libraries like Redux offer middleware and tools to help manage state securely and prevent unauthorized state modifications.
- Security Best Practices: The React community and documentation provide guidelines on secure coding practices, such as avoiding the use of eval and ensuring that third-party libraries are secure.
Best Practices:
- Avoid using dangerouslySetInnerHTML, or ensure that the injected content is sanitized.
- Implement CSP headers to control the sources of content that can be loaded into your application.
- Use secure state management practices with libraries like Redux.
- Regularly update React and its dependencies to include the latest security fixes.
Angular offers a more comprehensive set of built-in security features, such as automatic sanitization, SCE, and XSRF protection, which help developers build secure applications out-of-the-box.
React, while providing essential security features like JSX escaping and support for CSP, relies more on developers following best practices and using additional libraries to ensure security.
Both frameworks emphasize the importance of regular updates and secure coding practices to maintain application security.
Feature | Angular | Steeper initial learning curve due to TypeScript and its comprehensive nature |
Learning Curve | Steeper initial learning curve due to TypeScript and comprehensive nature | Gentler initial learning curve due to focus on UI components |
Syntax | TypeScript, decorators, templates | JavaScript (JSX), functional/class components |
Approach | Opinionated framework with MVVM architecture | Library with component-based architecture |
Performance | Potentially less performant for large applications due to two-way data binding | Generally better performance for dynamic interfaces due to virtual DOM |
Tooling | Angular CLI, strong IDE support, built-in testing tools | Create React App, good IDE support, relies on third-party tools |
Scalability | Modular architecture, TypeScript, dependency injection, enterprise-level support | Component-based architecture, flexibility, state management libraries |
Security Features | Built-in sanitization, Content Security Policy (CSP), HTTP Client security, Strict Contextual Escaping (SCE) | JSX escaping, CSP support, relies on best practices and third-party libraries |
Use Cases: When to Choose Angular & When to Choose React
Choosing between Angular and React depends on the specific requirements of your project as well as the expertise of your development team. Here are some scenarios that can help guide your decision.
When to Choose Angular
- Large, Enterprise-Level Applications: Angular’s comprehensive framework with built-in features like routing, form validation, and HTTP client makes it well-suited for large-scale applications that require a structured and maintainable architecture.
- Team Familiarity with TypeScript: If your team is proficient in TypeScript or willing to adopt it, Angular’s use of TypeScript can lead to more robust and maintainable code.
- Complex Projects with Multiple Dependencies: Its dependency injection and modular architecture make it easier to manage complex projects with multiple dependencies.
- Need for a Standardized Development Environment: Angular’s opinionated and standardized approach, along with its powerful CLI, can streamline the development process and ensure consistency across large teams.
- Real-Time Applications: Angular’s two-way data binding is particularly useful for real-time applications where the view needs to be updated frequently based on user interactions.
- Comprehensive Tooling: If you prefer a framework that comes with built-in tools for testing, debugging, and deployment, Angular’s CLI and integrated tools can be very beneficial.
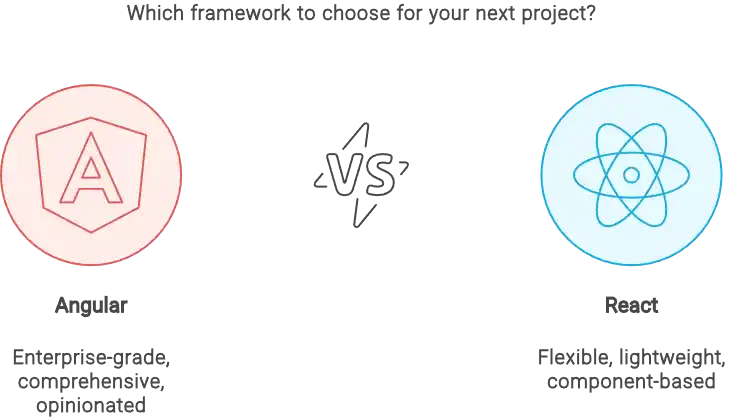
When to Choose React
- Dynamic and Interactive User Interfaces: React’s virtual DOM and efficient reconciliation algorithm make it ideal for applications with dynamic and interactive user interfaces that require frequent updates.
- Flexibility and Customizability: If you need a flexible, unopinionated library that allows you to choose your tools and libraries for state management, routing, etc., React’s modularity offers greater customizability.
- Existing JavaScript Proficiency: For teams with strong JavaScript skills, React’s use of JSX and JavaScript can make the learning curve gentler and development faster.
- Component Reusability: React’s component-based architecture promotes the creation of reusable components, making it easier to manage and scale applications.
- Cross-Platform Development: If you plan to develop both web and mobile applications, React’s ecosystem, including React Native for mobile development, allows you to use the same principles and components across platforms.
- Incremental Adoption: React can be incrementally adopted into existing projects, making it easier to integrate with other libraries or frameworks if you don’t want to rewrite your entire codebase.
Conclusion
Ultimately, the choice between Angular and React should be based on a thorough evaluation of your project requirements, team expertise, and long-term maintenance considerations.
By assessing the specific needs of your application and the strengths of each framework, you can make an informed decision that ensures the success of your project.
- Choose Angular for large, complex applications that benefit from a structured, all-in-one framework with built-in tools and TypeScript support.
- Choose React for dynamic, interactive applications that require flexibility, component reusability, and potential cross-platform development.
Evaluating these factors will help you determine the best fit for your project and ensure a smooth development process.