Table of Contents
In the world of web development, testing APIs, automating tasks, or downloading files from the internet are essential operations. One tool that makes these tasks incredibly simple and powerful is cURL
. Whether you’re a seasoned developer or someone just starting out, understanding how to use cURL will elevate your web-based projects.
In this blog, we’ll walk you through the basics and progressively dive deeper into cURL’s functionalities.
What is cURL?
cURL, short for Client URL, is a command-line tool that allows you to transfer data to or from a server. It works with various protocols, but it’s most commonly used with HTTP and HTTPS. It is used to communicate with web servers and send or receive information using URLs, making it ideal for interacting with APIs and web services.
Key Features:
- Multi-protocol support: HTTP, HTTPS, FTP, SMTP, and more.
- Ability to upload or download files.
- Test APIs or web services.
- Authentication and cookies support.
- Completely open-source and free to use.
Why Should You Use cURL?
cURL is a versatile and flexible tool. It can be used in scenarios like:
- Testing RESTful APIs during development.
- Fetching web pages and interacting with web servers.
- Automating file downloads or uploads.
- Debugging HTTP requests by checking the headers and response.
Next, we’ll break down how to use it step-by-step.
Getting Started
Let’s start with some basic operations you can perform using cURL. Here are a few simple commands that will help you get comfortable with the tool.
1. Fetching a Webpage
The simplest use of cURL is to fetch and display the contents of a webpage. You can do this by simply typing the following command:
curl https://example.com
For Example:
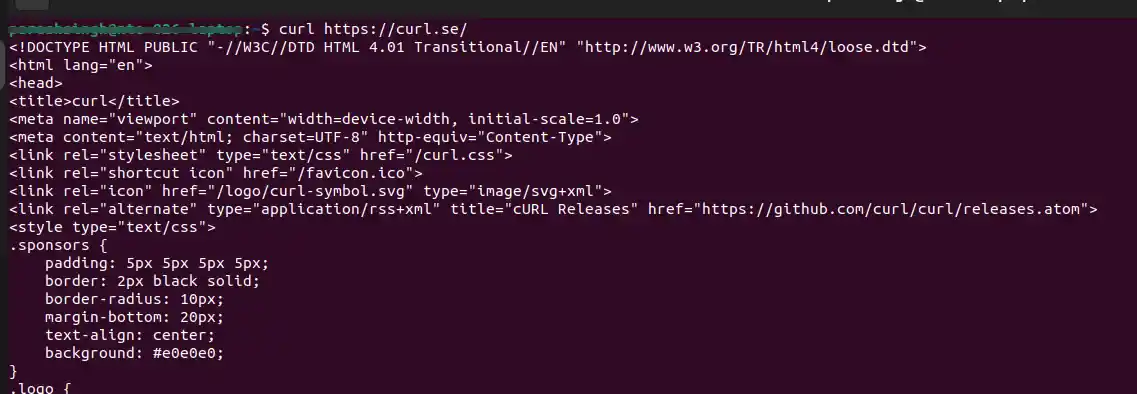
Explanation:
This command fetches the webpage at https://example.com
and displays its HTML content in the terminal. The ability to retrieve webpages is useful when you want to inspect a site’s structure or download raw data from the server.
2. Saving the Output to a File
To save the webpage content to a file instead of displaying it on the terminal, use the -o
option:
curl https://example.com -o output.html
Explanation:
Here, the file output.html
will contain the HTML source of the webpage. This is helpful if you want to download a file from the web or save the page’s content for later inspection.
Sending Data
cURL is more than just a file-fetching tool. It allows you to send data to servers, which is especially useful for interacting with APIs.
3. Sending a POST Request
To send data via POST (a common method in web development), use the -X POST
option:
curl -X POST https://example.com/api -d "param1=value1¶m2=value2"
Explanation:
In this example, the -d
option sends form data to the server. POST requests are commonly used for submitting data, such as filling in a form on a website or sending login credentials to an API.
Adding Custom Headers
Many web services require you to include custom headers with your requests. For example, APIs often use headers for authentication purposes.
4. Adding HTTP Headers
To include a custom header in your request, use the -H
option. A common use case is passing an API key or a Bearer Token:
curl -H "Authorization: Bearer your_token" https://example.com/api
Explanation:
This is useful when you are working with APIs that require authentication. The header allows you to send tokens, session IDs, or custom parameters to the server.
Handling File Downloads
One of cURL’s strengths is handling file downloads efficiently.
5. Downloading Files
To download a file from a URL, you can use the -O
option:
curl -O https://example.com/file.zip
Explanation:
This command downloads the file at https://example.com/file.zip
and saves it in your current directory with the same name. This feature is handy for batch downloading, backups, or retrieving files from servers programmatically.
6. Follow Redirects
Sometimes URLs will redirect you to another location. Use the -L
flag to ensure that Client URL follows the redirect:
curl -L https://example.com
Explanation:
This is especially important for URLs that use services like URL shortening or redirection-based login flows
Viewing HTTP Headers and Response
When you’re debugging API requests, it’s important to see the headers returned by the server. More on Http.
7. Checking Response Headers
To fetch only the headers of a response (useful for debugging or checking server response codes), use the -I
option:
curl -I https://example.com
Explanation:
This command fetches only the HTTP headers of the server’s response, such as the status code (200 OK
, 404 Not Found
), content type, and more.
8. Verbose Mode for Debugging
If you’re troubleshooting issues with a request, use the -v
(verbose) flag to get detailed information about the request and response:
curl -v https://example.com
Explanation:
Verbose mode outputs all the details, including the headers sent, the data being transferred, and any other information necessary for debugging.
Advanced Usage
Now that we’ve covered the basics, let’s explore more advanced use cases.
9. Uploading Files
You can use cURL to upload files via a POST request. This is useful for submitting files through APIs:
curl -F "file=@/path/to/file.zip" https://example.com/upload
Explanation:
In this example, the -F
flag uploads the specified file to the server. This method is widely used in APIs that allow file uploads.
10. Working with JSON Data
Many modern APIs require data in JSON format. You can easily send JSON data with Client URL:
curl -X POST https://example.com/api -H "Content-Type: application/json" -d '{"key1":"value1", "key2":"value2"}'
Explanation:
The -H
option sets the Content-Type
header to application/json
, and the -d
option sends the JSON payload.
Working with Authentication and Cookies
Many web services require authentication, and Client URL handles various methods of authentication, such as Basic, Bearer Token, and cookies.
11. Handling Authentication
You can use cURL to send requests that require authentication. Here’s how you’d send a request using Basic Authentication:
curl -u username:password https://example.com
For more secure APIs that require tokens, use the Bearer Token method:
curl -H "Authorization: Bearer your_token" https://example.com
12. Saving and Sending Cookies
You can save cookies from a request using the -c
option:
curl -c cookies.txt https://example.com
To send cookies back in future requests, use the -b
option:
curl -b cookies.txt https://example.com
This is helpful for session management when you’re interacting with websites that require login and cookie-based authentication.
Conclusion
cURL is an incredibly powerful tool that makes interacting with web services and APIs much simpler. From fetching data to sending files, it handles it all with ease. With features like custom headers, file uploads, JSON support, and authentication, you can use it for a wide variety of tasks in web development.
As you grow more comfortable with the basics, try automating workflows or integrating Client URL into your scripts to boost productivity. cURL’s flexibility, coupled with its robust feature set, makes it a must-have tool in every developer’s toolkit. Whether you’re a beginner or a pro, mastering it will help you communicate more effectively with the web.