Table of Contents
Golang, or simply Go, is a modern programming language that is recognized for its simplicity, efficiency, and rapid scalability. Developed by Google in 2009, it quickly gained popularity among developers dealing with web servers, cloud applications, or any other performance-hungry software.
This guide will walk you through the fundamental steps needed to get started with Golang-from installing software to writing your own first program. This simple instructive guide will ease the way for developers already experienced with other programming languages or first-time programmers to dive right in.
Why Learn This Language?
Golang model is Sandwiched right between simple and powerful, which has made it one of the choicest programming languages. Here are the reasons you might like to learn it:
- Simple Syntax: It has Clean code and a straightforward syntax Simple to learn, but still a great choice for beginners as well as a seasoned developer.
- High Performance: As a compiled language, It runs extremely fast; it can rival performance-heavy languages like C and C++.
- Concurrency: Built-in language concurrency with go routines makes it useful for scalable applications.
- Strong Community: A vibrant ecosystem offers libraries, frameworks, and tools to streamline development.
Setting Up Your Development Environment
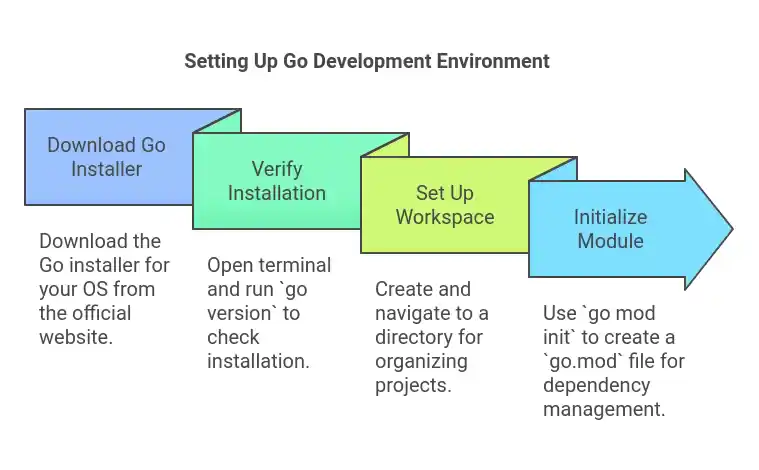
1. Install the Language: Download it from https://golang.org/dl/.
Download the installer for your operating system (Windows, macOS, or Linux) from the official website, and follow the provided instructions to complete the setup.
2. Verify Installation: Open up a terminal or command prompt and run:
go version
You should see the installed Go version.
3. Set Up Your Workspace: By default, projects are organized in a workspace. Set up a directory to organize your projects:
mkdir go-projects
cd go-projects
4. Tracking dependencies becomes: Your code will manage this with go.mod
file when it imports packages belonging to other modules. The file remembers the modules that are providing those packages.
To create a go.mod
file, you would need to use the command go mod init
. This will initialize your module and assign module path.
For example:
go mod init example/go-projects
Output:
go: creating new go.mod: module example/go-projects
Here the “example/go-projects” is the module path which oftentimes corresponds to where the repository will be containing your code.
Writing Your First Program
Create a File: Create a new file called main.go
in your project folder.
Write the Code:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!!!")
}
Run the Program: Golang to your command window, navigate to your project directory, and run:
go run main.go
Output:
Hello, World!!!
Understanding Core Concepts
Packages: Every Golang file starts off with a package declaration.
package main shows that this is an executable program.
Imports:
Using import allows you to bring in libraries like fmt for formatted I/O.
Main Functions:
Execution begins with the main() function, which serves as its entry point.
Variables:
var name string = "Go"
age := 10 // shorthand for declaring and initializing variables
Control Structures:
Loops:
for age := 0; age < 5; age++ {
fmt.Println(age)
}
Conditional Statements:
if age > 5 {
fmt.Println("Go is older than 5 years.")
}
Concurrency: Golang’s goroutines make it easy to run functions concurrently:
go fmt.Println("Running in a goroutine")
Building and compiling Your Code
To build and compile your Golang program into a standalone executable, run:
go build main.go
This will produce a binary that can be run without needing Go to be installed.
Conclusion
That’s basically a very powerful language for overcoming the challenges of modern programming. A clean syntax, a powerful concurrency model, and a rich ecosystem make it ideal both for beginners and professionals. You have taken the first step into the world of Go programming. Practice and exploration are what you will need to become a confident developer who builds scalable high-performance applications.