Table of Contents
The world of web development is constantly evolving, with users expecting increasingly interactive and engaging websites. One of the most powerful tools for creating smooth and professional animations is GSAP (GreenSock Animation Platform). It has become the go-to choice for developers looking to bring their designs to life without sacrificing performance. In this comprehensive guide, we’ll delve into the essentials of this, its features, and how to effectively use it to create stunning animations.
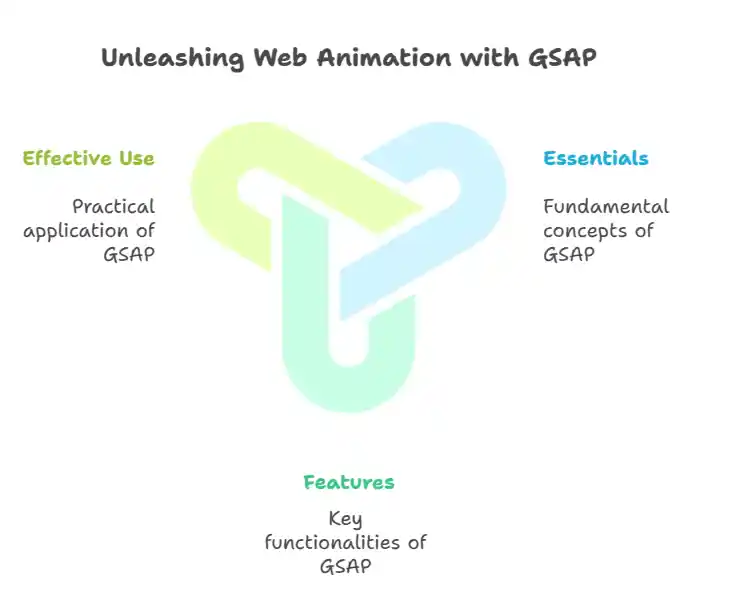
What is GreenSock Animation Platform?
It is a JavaScript library designed to create high-performance animations for the web. Whether you’re working with CSS, SVG, canvas, or WebGL, it provides robust tools to animate elements with precision and ease. Unlike other animation libraries, it prioritizes performance and compatibility, making it a preferred choice for both small projects and large-scale applications.
Key Features of GreenSock Animation Platform:
- Cross-browser compatibility: Ensures consistent animations across different browsers.
- High performance: Optimized for speed, It outperforms native CSS animations in many cases.
- Rich API: Offers a wide range of features for customizing animations.
- Plugins and extensibility: Comes with plugins like ScrollTrigger, MorphSVG, and more to enhance functionality.
- Ease of use: Simplifies complex animations with intuitive syntax.
- Backward compatibility: It ensures older browsers are supported, making it a reliable choice for various projects.
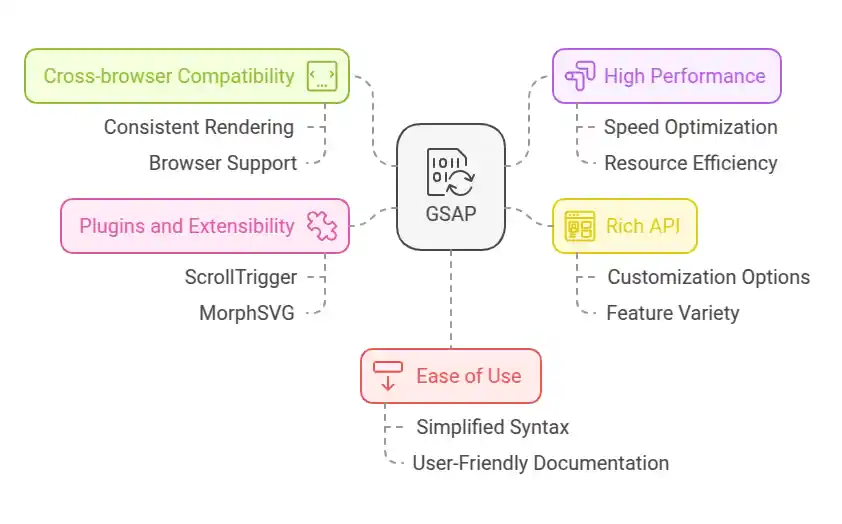
Why Use GreenSock Animation Platform Over CSS Animations?
While CSS animations are powerful and simple for basic tasks, It offers unmatched flexibility and control for advanced animations. Here’s how GreenSock Animation Platform stands out:
- Complex Sequences: Easily chain multiple animations together using timelines.
- Dynamic Animations: Adjust properties dynamically based on user interactions.
- Precision: Animate any property, including non-CSS properties like
scrollTop
or canvas properties. - Extensibility: The plugin system makes it easy to achieve effects that CSS alone cannot handle, like morphing SVGs or scroll-based triggers.
- Better Performance: It optimizes animations to reduce layout thrashing and GPU usage, ensuring smoother playback.
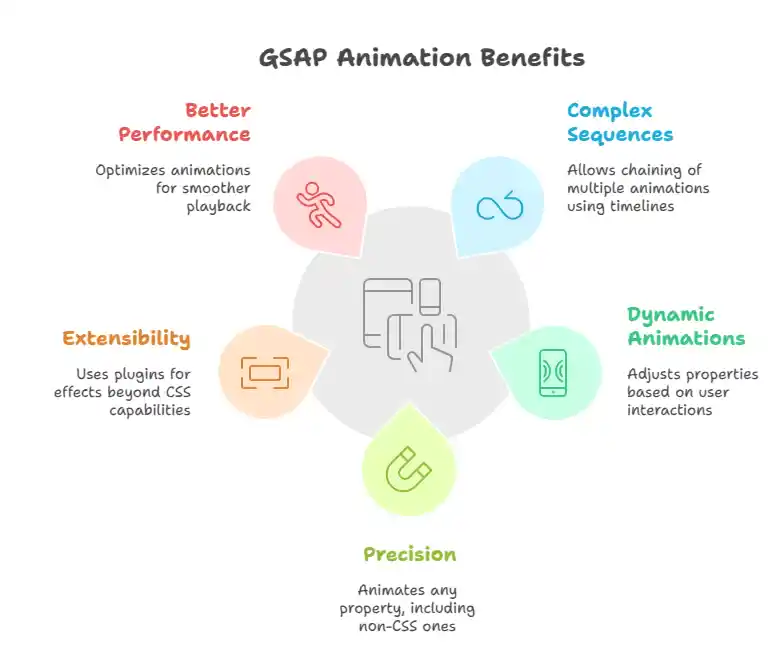
Getting Started with GreenSock Animation Platform
To begin using it, you need to include the library in your project. You can either download it or use a CDN link.
Installation:
Using npm:
npm install gsap
Using a CDN:
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.2/gsap.min.js"></script>
Basic Example:
<div class="box"></div>
<style>
.box {
width: 100px;
height: 100px;
background-color: blue;
margin: 50px auto;
}
</style>
<script>
gsap.to(".box", {
duration: 2,
x: 200,
backgroundColor: "red",
scale: 1.5
});
</script>
This code moves the .box
element 200px to the right, changes its background color to red, and scales it up by 1.5 times over 2 seconds.
Basic Syntax:
GreenSock Animation Platform animations revolve around the gsap.to()
, gsap.from()
, and gsap.fromTo()
methods.
Example:
// Animate an element to change its opacity and x position
gsap.to(".box", {
duration: 1,
x: 100,
opacity: 0.5
});
Advanced Example: ScrollTrigger with Timelines
<div class="container">
<div class="box"></div>
</div>
<style>
.container {
height: 200vh; /* For scroll effect */
display: flex;
align-items: center;
justify-content: center;
}
.box {
width: 100px;
height: 100px;
background-color: blue;
}
</style>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.2/gsap.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.2/ScrollTrigger.min.js"></script>
<script>
gsap.registerPlugin(ScrollTrigger);
const tl = gsap.timeline({
scrollTrigger: {
trigger: ".container",
start: "top center",
end: "bottom center",
scrub: true,
},
});
tl.to(".box", { x: 300, rotation: 180, duration: 2 })
.to(".box", { backgroundColor: "red", scale: 2, duration: 1 });
</script>
Core Concepts of GreenSock Animation Platform
Tween
A “tween” refers to a single animation instance in it. For example, animating the position of a box is a tween.
Tween Example:
// Move an element vertically
gsap.to(".circle", {
duration: 2,
y: 150
});
This simple tween animates the .circle
element downward by 150px in 2 seconds.
Timeline
A timeline allows you to sequence multiple tweens, making it easier to create complex animations with precise timing.
Example:
const tl = gsap.timeline();
// Add tweens to the timeline
tl.to(".box1", { duration: 1, x: 100 })
.to(".box2", { duration: 1, y: 100 })
.to(".box3", { duration: 1, rotation: 360 });
This timeline sequentially animates three elements. The .box1
moves along the x-axis, .box2
moves along the y-axis, and .box3
rotates 360 degrees.
Easing
GreenSock Animation Platform offers a variety of easing functions to control the rate of change of your animations. Popular easing options include power1
, power2
, bounce
, and elastic
.
Example:
gsap.to(".circle", {
duration: 2,
y: 200,
ease: "bounce.out"
});
This example uses the bounce.out
easing, causing the animation to mimic a bouncing effect as it slows down near the end.
Looping Animations
GreenSock Animation Platform makes it simple to create looping animations.
Example:
gsap.to(".spinner", {
duration: 1,
rotation: 360,
repeat: -1,
ease: "linear"
});
Here, the .spinner
element rotates endlessly with a linear easing.
Popular GreenSock Animation Platform Plugins
GSAP’s plugin ecosystem extends its capabilities, making it even more powerful. Below are some of the most popular plugins:
ScrollTrigger
This plugin enables animations triggered by scroll events. It’s ideal for creating parallax effects, revealing content on scroll, and more.
Example:
// Animate an element when it comes into view
gsap.to(".section", {
scrollTrigger: {
trigger: ".section",
start: "top center",
end: "bottom center",
toggleActions: "play none none reverse",
},
opacity: 1,
y: 0,
});
This example sets up a scroll-triggered animation that fades in and moves a .section
element upward when it enters the viewport.
Parallax Effect Example:
gsap.to(".background", {
y: -200,
scrollTrigger: {
trigger: ".container",
start: "top bottom",
scrub: true,
},
});
Advanced ScrollTrigger Example:
gsap.to(".image", {
scrollTrigger: {
trigger: ".image-container",
start: "top bottom",
end: "bottom top",
scrub: true
},
scale: 1.5
});
This animation scales an .image
element as the user scrolls through its parent container.
MorphSVG
The MorphSVG plugin lets you morph SVG shapes seamlessly.
Example:
// Morph one SVG shape into another
gsap.to("#circle", {
duration: 2,
morphSVG: "#star"
});
This morphs a circular SVG into a star shape, providing visually appealing transitions between SVG elements.
SplitText
SplitText makes it easy to animate individual letters, words, or lines of text.
Example:
const split = new SplitText(".headline", { type: "words" });
gsap.to(split.words, {
duration: 1,
opacity: 1,
stagger: 0.1
});
This morphs a circular SVG into a star shape, providing visually appealing transitions between SVG elements.
MotionPathPlugin
Animate elements along a defined path.
Example:
gsap.to(".plane", {
duration: 5,
motionPath: {
path: "#path",
align: "#path",
alignOrigin: [0.5, 0.5]
}
});
This example animates a .plane
element along an SVG path defined by the #path
element.
Draggable
Draggable.create(".box", {
type: "x,y",
bounds: "#container",
onDragEnd: function () {
console.log("Drag ended!");
},
});
Best Practices for GreenSock Animation Platform Animation
1. Optimize Performance
- Use
will-change
CSS property for smoother animations. - Avoid animating large DOM elements whenever possible.
- Reduce the number of simultaneous animations.
2. Plan Your Animations
Before coding, sketch out your animation sequence to ensure clarity and efficiency.
3. Use Timelines for Complex Animations
Timelines provide better control over multiple tweens, ensuring a cohesive flow.
4. Debug with GSDevTools
GSDevTools is a plugin that helps you debug animations visually, making it easier to fine-tune your animations.
5. Avoid Overloading Animations
Too many animations can overwhelm users and impact performance. Prioritize meaningful animations that enhance the user experience.
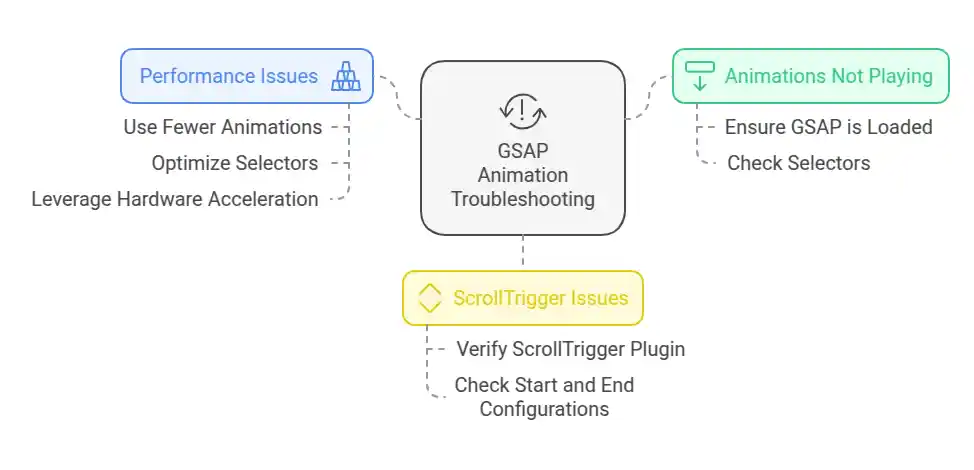
Advanced Techniques with GreenSock Animation Platform
1. Responsive Animations
Create animations that adapt to different screen sizes using media queries.
Example:
gsap.to(".box", {
duration: 1,
x: 100,
scrollTrigger: {
trigger: ".box",
start: "top center",
end: "bottom center",
toggleActions: "play reverse play reverse"
}
});
This animation triggers as the .box
element scrolls into view and adapts dynamically to screen sizes.
2. Staggered Animations
Apply animations to multiple elements with staggered timing.
Example:
gsap.to(".items", {
duration: 1,
y: 50,
stagger: 0.2
});
This example animates a series of .items
elements with a 0.2-second delay between each.
3. Custom Easing
Define your own easing curves for unique motion effects.
Example:
gsap.to(".ball", {
duration: 2,
x: 300,
ease: "custom"
});
Custom easing allows for more creative and dynamic animations tailored to your project needs.
4. Combining ScrollTrigger and Timelines
Use ScrollTrigger with timelines for scroll-based animation sequences.
Example:
const tl = gsap.timeline({
scrollTrigger: {
trigger: ".container",
start: "top top",
end: "bottom bottom",
scrub: true
}
});
tl.to(".box", { x: 200, duration: 1 })
.to(".circle", { y: 150, duration: 1 });
This example combines timeline animations with scrolling, creating a synchronized
Troubleshooting Common Issues
1. Animations Not Playing
- Ensure the GreenSock Animation Platform library is correctly loaded.
- Check the selectors and confirm they match the DOM elements.
2. Performance Issues
- Use fewer animations simultaneously.
- Optimize selectors and DOM structure.
- Leverage hardware acceleration.
3. Debugging Tips
- Use console logs to confirm elements and properties.
- Verify that plugins are loaded if using advanced features like ScrollTrigger.
- Experiment with
GSDevTools
for real-time debugging of timelines and tweens.
4. ScrollTrigger Issues
- Verify ScrollTrigger plugin is correctly included.
- Check the
start
andend
configurations.
GreenSock Animation Platform Community and Resources
It has an active community that offers a wealth of resources to help developers at all levels:
- GreenSock Animation Platform Documentation: The official GSAP docs provide detailed explanations and examples.
- GreenSock Forums: A vibrant forum where you can ask questions and share your projects.
- CodePen: Search for this examples on CodePen for inspiration and reusable snippets.
Pros and Cons of GreenSock Animation Platform
Pros:
- Unparalleled Performance
It is optimized for speed, often outperforming native CSS animations, especially for complex or large-scale projects. - Cross-Browser Compatibility
It ensures that your animations work consistently across all major browsers, including older versions. - Rich API
It offers an intuitive API with methods liketo()
,from()
, andtimeline()
that simplify complex animations. - Advanced Plugins
Plugins like ScrollTrigger, Draggable, and MorphSVG add unique capabilities, making GreenSock Animation Platform extremely versatile. - Extensive Community Support
It has an active community, comprehensive documentation, and a wealth of tutorials and examples. - Versatility
It works seamlessly with CSS, JavaScript, SVG, canvas, and WebGL, offering endless animation possibilities. - Customizable Easing Functions
It provides precise control over easing, enabling unique animation effects.
Cons:
- Learning Curve
Beginners might find it overwhelming due to its extensive features and flexibility. - File Size
While lightweight for its capabilities, it is larger than native CSS animations or simpler libraries, which may impact initial load time in smaller projects. - Dependency on JavaScript
GreenSock Animation Platform animations won’t work if JavaScript is disabled in the user’s browser. - Cost for Premium Plugins
While GreenSock Animation Platform is free for most features, some advanced plugins like MorphSVG and SplitText require a paid license for commercial use.
GSAP vs. CSS Animations
Feature | GreenSock Animation Platform | CSS Animations |
---|
Performance | Optimized for speed and complex interactions | Suitable for basic animations |
Ease of Use | Intuitive API but requires JavaScript knowledge | Suitable for basic animations |
Flexibility | Works with CSS, SVG, WebGL, and canvas | Limited to CSS properties |
Plugins | Rich plugin ecosystem | No plugin support |
Browser Support | Excellent, including older browsers | Limited in older browsers |
Conclusion
GreenSock Animation Platform is a versatile and powerful tool that simplifies the process of creating stunning web animations. Whether you’re a beginner or an experienced developer, mastering GSAP can elevate your web development skills and help you deliver engaging user experiences. By following the techniques and best practices outlined in this guide, you’ll be well-equipped to harness the full potential of GSAP.
Start experimenting with GreenSock Animation Platform today and transform your ideas into visually captivating animations that leave a lasting impression on your users!
READ MORE:
Mastering Mobile Responsiveness: Building Websites That Stunning on Any Device
Effective AI in Hospitals: Reducing Burnout and Maximizing Efficiency