Table of Contents
Laravel is a powerful PHP framework that simplifies web application development. While CRUD (Create, Read, Update, Delete) operations are a great starting point for beginners, real-world applications often demand complex database interactions. To create scalable and maintainable systems, developers need to harness the full potential of Laravel’s robust ORM (Eloquent) for implementing intricate relationships.
In this blog, we will explore how to move beyond CRUD by delving into advanced database relationships in Laravel. By the end, you’ll gain insights into modeling and querying these relationships effectively.
Why Go Beyond CRUD?
CRUD operations are the foundation of most applications. However, as applications grow in complexity, so do the relationships between data entities. For instance, a blogging platform may require features like user roles, post categories, and multimedia attachments, which involve more than simple CRUD operations. Understanding these advanced relationships allows you to:
- Optimize Performance: Efficiently retrieve and manipulate data.
- Enhance Maintainability: Write cleaner, reusable code.
- Build Scalable Systems: Support complex business logic.
Moreover, advanced relationships reduce code duplication, ensure data consistency, and enable developers to integrate complex workflows seamlessly.
One-to-Many Relationships
A one-to-many relationship is when one record in a table relates to multiple records in another table. For example, a Post
model may have many Comment
models, but each comment belongs to a single post.
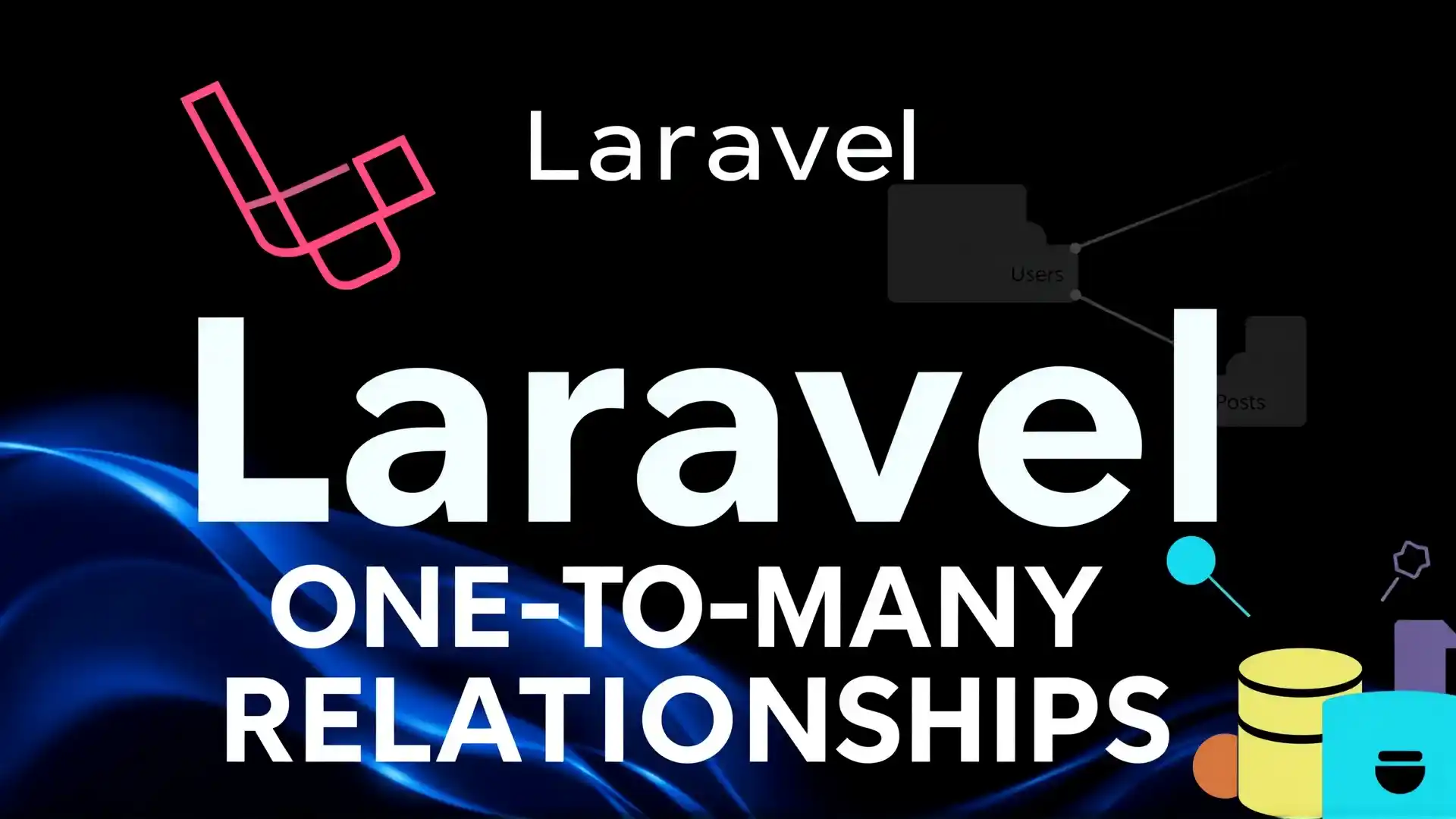
Setting Up the Models
Here’s how you can define this relationship in Laravel:
// app/Models/Post.php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
public function comments()
{
return $this->hasMany(Comment::class);
}
}
// app/Models/Comment.php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Comment extends Model
{
public function post()
{
return $this->belongsTo(Post::class);
}
}
Querying the Relationship
Retrieve all comments for a post:
$post = Post::find(1);
$comments = $post->comments;
Retrieve the post for a comment:
$comment = Comment::find(1);
$post = $comment->post;
Using Eager Loading
Eager loading reduces the number of queries executed:
$posts = Post::with('comments')->get();
This ensures that related comments are fetched in a single query, improving performance when dealing with large datasets.
Real-World Scenario
Imagine building a forum application where each thread has multiple replies. The one-to-many relationship ensures replies are efficiently linked to their parent threads, enabling features like nested discussions and reply counts.
Many-to-Many Relationships
Many-to-many relationships occur when multiple records in one table relate to multiple records in another table. For example, User
and Role
models can have a many-to-many relationship since a user can have multiple roles and a role can belong to multiple users.
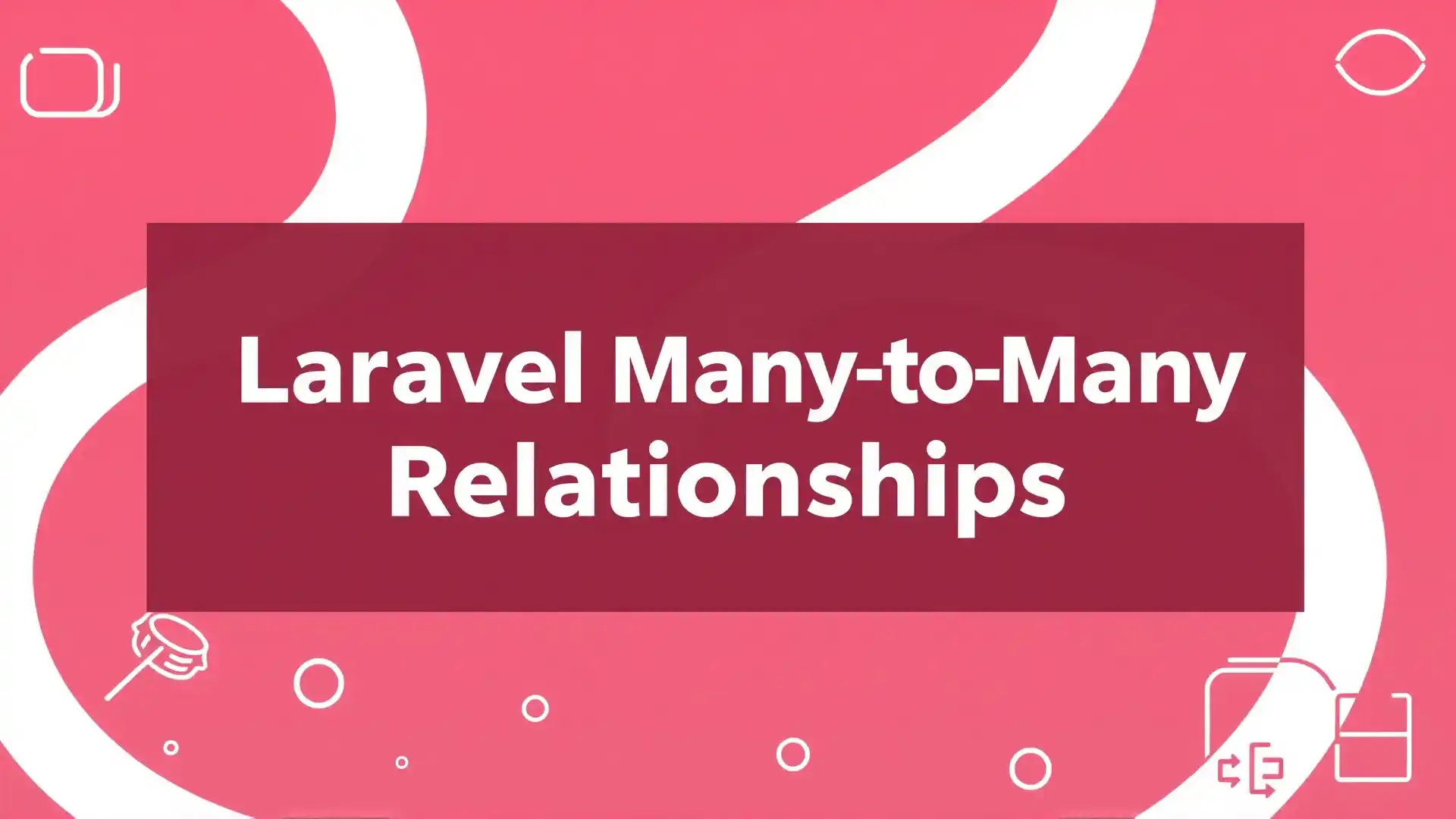
Setting Up the Models
Create a pivot table to manage the relationship:
Schema::create('role_user', function (Blueprint $table) {
$table->id();
$table->foreignId('user_id')->constrained()->onDelete('cascade');
$table->foreignId('role_id')->constrained()->onDelete('cascade');
$table->timestamps();
});
Define the relationships in the models:
// app/Models/User.php
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class);
}
}
// app/Models/Role.php
class Role extends Model
{
public function users()
{
return $this->belongsToMany(User::class);
}
}
Managing the Relationship
Attach roles to a user:
$user = User::find(1);
$user->roles()->attach([1, 2]);
Detach roles:
$user->roles()->detach(2);
Sync roles:
$user->roles()->sync([1, 3]);
Retrieve users with specific roles using eager loading:
$users = User::with('roles')->whereHas('roles', function ($query) {
$query->where('name', 'Admin');
})->get();
Real-World Scenario
Consider an e-commerce application where products can belong to multiple categories, and categories can have multiple products. This many-to-many relationship allows dynamic categorization and efficient retrieval of products by category.
Polymorphic Relationships
Polymorphic relationships allow a model to belong to more than one type of model using a single association. For example, an Image
model can be associated with both Post
and User
models.
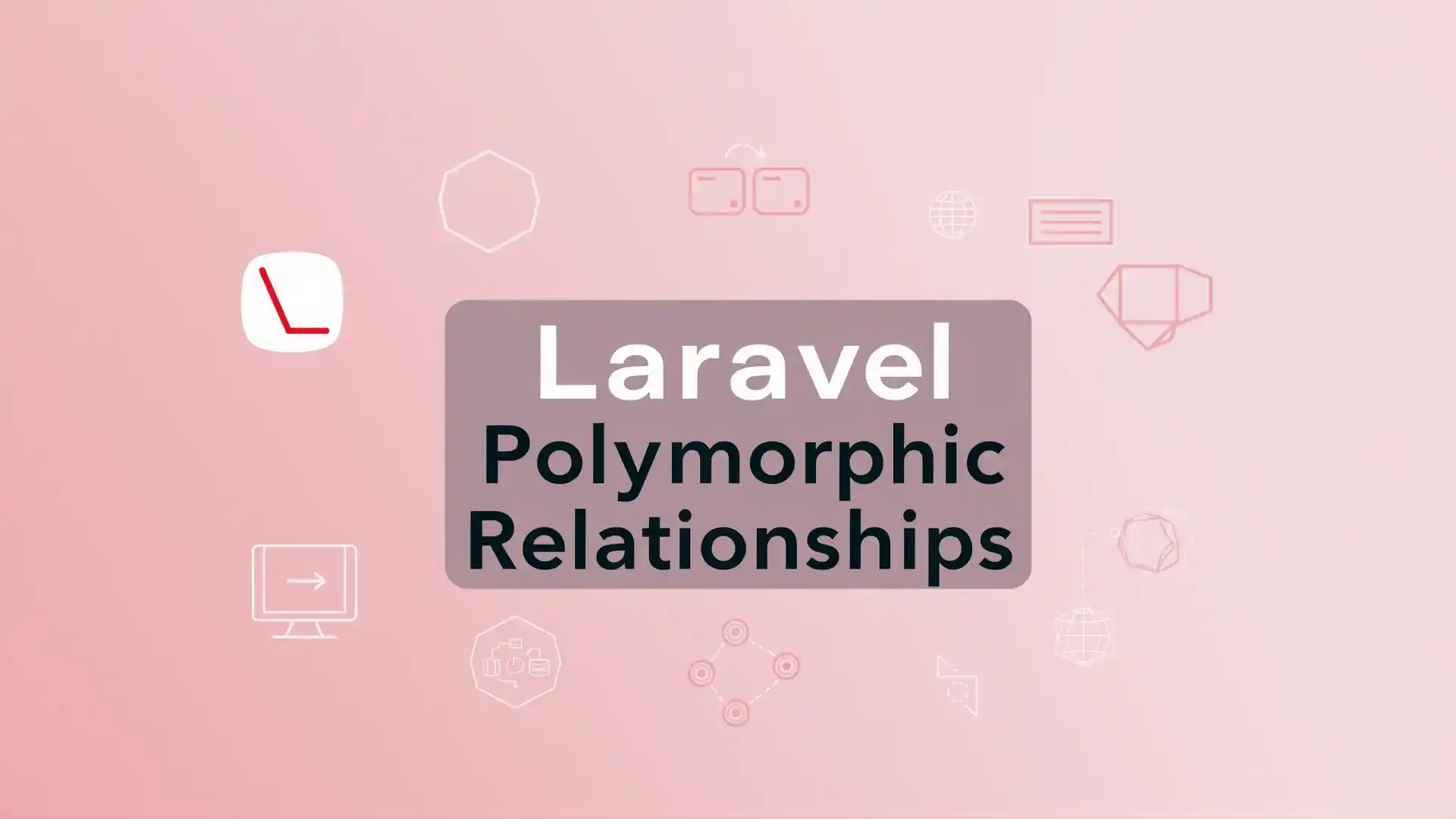
Setting Up the Models
Define the polymorphic relationships:
// app/Models/Image.php
class Image extends Model
{
public function imageable()
{
return $this->morphTo();
}
}
// app/Models/Post.php
class Post extends Model
{
public function images()
{
return $this->morphMany(Image::class, 'imageable');
}
}
// app/Models/User.php
class User extends Model
{
public function images()
{
return $this->morphMany(Image::class, 'imageable');
}
}
Querying Polymorphic Relationships
Retrieve images for a post:
$post = Post::find(1);
$images = $post->images;
Retrieve the parent of an image:
$image = Image::find(1);
$imageable = $image->imageable;
Real-World Scenario
Polymorphic relationships are ideal for handling media attachments. For instance, in a CMS, images can be attached to blog posts, user profiles, or product listings, ensuring flexibility and scalability.
Advanced Querying Techniques
Laravel’s ORM provides advanced tools for querying complex relationships. Here are some examples:
Filtering Related Data
Retrieve posts with comments containing specific keywords:
$posts = Post::whereHas('comments', function ($query) {
$query->where('content', 'like', '%Laravel%');
})->get();
Counting Related Records
Retrieve posts with more than 5 comments:
$posts = Post::has('comments', '>', 5)->get();
Aggregating Data
Calculate the average rating for posts based on related reviews:
$posts = Post::withAvg('reviews', 'rating')->get();
Real-World Use Cases
- E-Commerce Systems: Use many-to-many relationships to manage product categories and tags.
- Content Management: Implement polymorphic relationships for handling attachments like images or videos.
- User Permissions: Define complex roles and permissions using pivot tables.
Tips for Optimizing Relationships
- Eager Loading: Use
with
to load related models upfront and reduce query counts. - Indexing: Ensure foreign key columns are indexed for better performance.
- Caching: Use caching mechanisms like Redis to store frequently accessed data.
- Chunking: Process large datasets in chunks to save memory:
Post::chunk(100, function ($posts) {
foreach ($posts as $post) {
// Process each post
}
});
- Database Normalization: Ensure tables are normalized to eliminate redundant data and maintain consistency.
Best Practices for Designing Relationships
- Understand Business Logic: Model relationships that accurately reflect the application’s real-world requirements.
- Document Relationships: Clearly document relationships in your codebase for maintainability.
- Use Descriptive Names: Name relationship methods intuitively (e.g.,
comments
instead ofdata
). - Test Relationships: Write tests to verify the integrity and correctness of relationships.
Conclusion
Implementing complex database relationships in Laravel unlocks a wealth of possibilities for building feature-rich and high-performing applications. From one-to-many and many-to-many to polymorphic relationships, Laravel’s ORM provides all the tools you need to model your data effectively.
By mastering these techniques, you can go beyond CRUD operations and build scalable applications that handle intricate business logic effortlessly.
Explore Laravel’s documentation further and practice these relationships in your projects to elevate your development skills. Remember, a well-structured database is the backbone of any successful application!